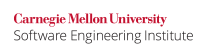
...
Code Block | ||
---|---|---|
| ||
class Dimensions { private int length; private int width; private int height; static public final int PADDING = 2; static public final int MAX_DIMENSION = 10; public Dimensions(int length, int width, int height) { this.length = length; this.width = width; this.height = height; } protected int getVolumePackage(int weight) { length += PADDING; width += PADDING; height += PADDING; try { if (length <= PADDING || width <= PADDING || height <= PADDING || length > MAX_DIMENSION + PADDING || width > MAX_DIMENSION + PADDING || height > MAX_DIMENSION + PADDING || weight <= 0 || weight > 20) { throw new IllegalArgumentException(); } int volume = length * width * height; // 12 * 12 * 12 = 1728 length -= PADDING; width -= PADDING; height -= PADDING; // Revert return volume; } catch (Throwable t) { MyExceptionReporter mer = new MyExceptionReporter(); mer.report(t); // Sanitize return -1; // Non-positive error code } } public static void main(String[] args) { Dimensions d = new Dimensions(8, 8, 8); System.out.println(d.getVolumePackage(21)); // Prints -1 (error) System.out.println(d.getVolumePackage(19)); // Prints 1728 2744(12x12x12) instead of 1000 1728(10x10x10) } } |
The catch
clause is permitted by exception ERR08-J-EX0 in ERR08-J. Do not catch NullPointerException or any of its ancestors because it serves as a general filter passing exceptions to the MyExceptionReporter
class, which is dedicated to safely reporting exceptions as recommended by ERR00-J. Do not suppress or ignore checked exceptions. Although this code only throws IllegalArgumentException
, the catch
clause is general enough to handle any exception in case the try
block should be modified to throw other exceptions.
...
Code Block | ||
---|---|---|
| ||
protected int getVolumePackage(int weight) {
length += PADDING;
width += PADDING;
height += PADDING;
try {
if (length <= PADDING || width <= PADDING || height <= PADDING ||
length > MAX_DIMENSION + PADDING ||
width > MAX_DIMENSION + PADDING ||
height > MAX_DIMENSION + PADDING ||
weight <= 0 || weight > 20) {
throw new IllegalArgumentException();
}
int volume = length * width * height; // 12 * 12 * 12 = 1728
return volume;
} catch (Throwable t) {
MyExceptionReporter mer = new MyExceptionReporter();
mer.report(t); // Sanitize
return -1; // Non-positive error code
} finally {
// Revert
length -= PADDING; width -= PADDING; height -= PADDING;
}
}
|
...