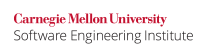
...
The C Standard, 6.5.7, paragraph 4 [ISO/IEC 9899:2011], states
...
In almost every case, an attempt to shift by a negative number of bits or by more bits than exist in the operand indicates a logic error. These issues are covered by INT34-C. Do not shift an expression by a negative number of bits or by greater than or equal to the number of bits that exist in the operand.
Noncompliant Code Example
This noncompliant code example performs a left shift, after verifying that the number being shifted is not negative, and the number of bits to shift is valid. The PRECISION()
macro and popcount()
function provide the correct precision for any integer type. (See INT35-C. Use correct integer precisions.) However, because this code does no overflow check, it can result in an unrepresentable value.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h>
#include <stddef.h>
#include <inttypes.h>
extern size_t popcount(uintmax_t);
#define PRECISION(umax_value) popcount(umax_value)
void func(signed long si_a, signed long si_b) {
signed long result;
if ((si_a < 0) || (si_b < 0) ||
(si_b >= PRECISION(ULONG_MAX))) {
/* Handle error */
} else {
result = si_a << si_b;
}
/* ... */
} |
Compliant Solution
This compliant solution eliminates the possibility of overflow resulting from a left-shift operation:
...