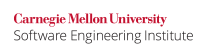
The java.security.AccessController
class is part of Java's security mechanism; it is responsible for enforcing the applicable security policy. This class's static doPrivileged()
method executes a code block with a relaxed security policy. The doPrivileged()
method stops permissions from being checked further down the call chain.
...
For example, suppose that a web application must maintain a sensitive password file for a web service and also must run untrusted code. The application could then enforce a security policy preventing the majority of its own code – as code—as well as all untrusted code – from code—from accessing the sensitive file. Because it must also provide mechanisms for adding and changing passwords, it can call the doPrivileged()
method to temporarily allow untrusted code to access the sensitive file. In this case, any privileged block must prevent any all information about passwords from being accessible to untrusted code.
...
Code Block | ||
---|---|---|
| ||
public class PasswordManager { public static void changePassword() throws FileNotFoundException { FileInputStream fin = openPasswordFile(); // testTest old password with password in file contents; change password, // then close the password file } public static FileInputStream openPasswordFile() throws FileNotFoundException { final String password_file = "password"; FileInputStream fin = null; try { fin = AccessController.doPrivileged( new PrivilegedExceptionAction<FileInputStream>() { public FileInputStream run() throws FileNotFoundException { // Sensitive action; can't be done outside privileged block FileInputStream in = new FileInputStream(password_file); return in; } }); } catch (PrivilegedActionException x) { Exception cause = x.getException(); if (cause instanceof FileNotFoundException) { throw (FileNotFoundException) cause; } else { throw new Error("Unexpected exception type", cause); } } return fin; } } |
...
In general, when any method containing a privileged block exposes a field (such as an object reference) beyond its own boundary, it becomes trivial for untrusted callers to exploit the program. This compliant solution mitigates the vulnerability by declaring openPasswordFile()
to be private. Consequently, an untrusted caller can call changePassword()
but cannot directly invoke the openPasswordFile()
method.
...
Compliant Solution (Hiding Exceptions)
Both the The previous noncompliant code example and the previous compliant solution throw a FileNotFoundException
when the password file is missing. If the existence of the password file is itself considered sensitive information, this exception also must also not be allowed to leak be prevented from leaking outside the trusted code.
This compliant solution suppresses the exception, leaving the array to contain a single null value to indicate that the file does not exist. It uses the simpler PrivilegedAction
class rather than PrivilegedExceptionAction
to prevent exceptions from propagating out of the doPrivileged()
block. The Void
return type is recommended for privileged actions that do not return any value.
Code Block | ||
---|---|---|
| ||
class PasswordManager { public static void changePassword() { FileInputStream fin = openPasswordFile(); if (fin == null) { // noNo password file; handle error } // testTest old password with password in file contents; change password } private static FileInputStream openPasswordFile() { final String password_file = "password"; final FileInputStream fin[] = { null }; AccessController.doPrivileged(new PrivilegedAction<Void>() { public Void run() { try { // Sensitive action; can't be done outside // doPrivileged() block fin[0] = new FileInputStream(password_file); } catch (FileNotFoundException x) { // reportReport to handler } return null; } }); return fin[0]; } } |
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SEC00-J | mediumMedium | likelyLikely | highHigh | P6 | L2 |
Automated Detection
Identifying sensitive information requires assistance from the programmer; fully automated identification of sensitive information is beyond the current state of the art.
...
Related Guidelines
CWE-266. Incorrect privilege assignment , Incorrect Privilege Assignment | |
Secure Coding Guidelines for the Java Programming LanguageSE, Version 35.0 | Guideline 6-2. 9-3 / ACCESS-3: Safely invoke |
Android Implementation Details
The java.security
package exists on Android for compatibility purposes only, and it should not be used.
Bibliography
Sections Section 6.4, " |
...