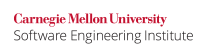
The C Standard, 6.7.3, paragraph 6 [ISO/IEC 9899:2011], states
If an attempt is made to modify an object defined with a
const
-qualified type through use of an lvalue with non-const
-qualified type, the behavior is undefined.
See also undefined behavior 64.
There are existing compiler implementations that allow const
-qualified objects to be modified without generating a warning message.
Avoid casting away const
qualification because doing so makes it possible to modify const
-qualified objects without issuing diagnostics. (See EXP05-C. Do not cast away a const qualification and STR30-C. Do not attempt to modify string literals for more details.)
Noncompliant Code Example
This noncompliant code example allows a constant object to be modified:
const int **ipp; int *ip; const int i = 42; void func(void) { ipp = &ip; /* Constraint violation */ *ipp = &i; /* Valid */ *ip = 0; /* Modifies constant i (was 42) */ }
The first assignment is unsafe because it allows the code that follows it to attempt to change the value of the const
object i
.
Implementation Details
If ipp
,
, and ip
i
are declared as automatic variables, this example compiles without warning with Microsoft Visual Studio 2013 when compiled in C mode (/TC
) and the resulting program changes the value of i
. GCC 4.8.1 generates a warning but compiles, and the resulting program changes the value of i
.
If ipp
, ip
, and i
are declared with static storage duration, this program compiles without warning and terminates abnormally with Microsoft Visual Studio 2013, and compiles with warning and terminates abnormally with GCC 4.8.1.
Compliant Solution
The compliant solution depends on the intent of the programmer. If the intent is that the value of i
is modifiable, then it should not be declared as a constant, as in this compliant solution:
int **ipp; int *ip; int i = 42; void func(void) { ipp = &ip; /* Valid */ *ipp = &i; /* Valid */ *ip = 0; /* Valid */ }
If the intent is that the value of i is not meant to change, then do not write noncompliant code that attempts to modify it.
Risk Assessment
Modifying constant objects through nonconstant references is undefined behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP40-C | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Astrée | 24.04 | assignment-to-non-modifiable-lvalue pointer-qualifier-cast-const pointer-qualifier-cast-const-implicit write-to-constant-memory | Fully checked |
Axivion Bauhaus Suite | 7.2.0 | CertC-EXP40 | |
Coverity | 2017.07 | PW MISRA C 2004 Rule 11.5 | Implemented |
Helix QAC | 2024.1 | C0563 | |
LDRA tool suite | 9.7.1 | 582 S | Fully implemented |
Parasoft C/C++test | 2023.1 | CERT_C-EXP40-a | A cast shall not remove any 'const' or 'volatile' qualification from the type of a pointer or reference |
Polyspace Bug Finder | R2024a | CERT C: Rule EXP40-C | Checks for write operations on const qualified objects (rule fully covered) |
RuleChecker | 24.04 | assignment-to-non-modifiable-lvalue pointer-qualifier-cast-const pointer-qualifier-cast-const-implicit | Partially checked |
TrustInSoft Analyzer | 1.38 | mem_access | Exhaustively verified (see the compliant and the non-compliant example). |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Key here (explains table format and definitions)
Taxonomy | Taxonomy item | Relationship |
---|---|---|
CERT C Secure Coding Standard | EXP05-C. Do not cast away a const qualification | Prior to 2018-01-12: CERT: Unspecified Relationship |
CERT C Secure Coding Standard | STR30-C. Do not attempt to modify string literals | Prior to 2018-01-12: CERT: Unspecified Relationship |
Bibliography
[ISO/IEC 9899:2011] | Subclause 6.7.3, "Type Qualifiers" |
11 Comments
Ron Natalie
This is entirely spurious. The posted example is NOT LEGAL C. The compiler will not allow the line marked CONSTRAING VIOLATION. There is nothing here that is not handled by the "DON'T CAST AWAY CONST" recommendation as a cast would have to be added to cause the above to be compilable.
Robert Seacord
This example will certainly compile... it even compiles without warning on MS VS with /W4.
It is also not the same as do not cast away const, because there is no cast used in the example.
Abhijit Rao
With MS VS 2008 - it generates an error - here is the portion of the error message
It does not matter what the Warning level is.
I have a general suggestion - regarding the box (panel) that contains the code (NCCE or CS), is it possible that these boxes can be made to display the line number in them ? Thank you
Stephen Friedl
I agree that this is spurious: not even MSVC6 compiles this code.
Robert Seacord
We may want to eliminate this rule.
Piotr Krukowiecki
VS compiles this code, even 8.0, depends on VS version. Whether anyone is using that versions is a different story.
David Svoboda
This rule is dead..we decided it was unsustainable.
You might look at 03. Expressions (EXP) for rules we still consider valid and supply comments there.
Martin Sebor
As discussed in the thread starting with this comment in EXP05-C. Do not cast away a const qualification, this rule is valid and valuable despite there being non-compliant examples that conforming implementations accept and generate code that runs with no adverse effects. For instance, the well-formed but non-compliant example below abends with
SIGSEGV
when compiled with gcc on Linux x64. I will go ahead and restore this rule.Martin Sebor
Restored to its original glory pursuant to the discussion Re: EXP05-C. Do not cast away a const qualification.
Martin Sebor
Values cannot be modified. This rule should be renamed to Do not modify constant objects.
Aaron Ballman
The assertion that Visual Studio warns is incorrect – it only warns if compiling in C++ mode (/TP). When compiling in C mode (/TC) it never warns, even at the highest warning levels.