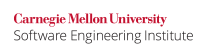
C99 provides the following definition of the asctime()
function in Section 7.23.3.1:
char *asctime(const struct tm *timeptr) { static const char wday_name[7][3] = { "Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat" }; static const char mon_name[12][3] = { "Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec" }; static char result[26]; sprintf(result, "%.3s %.3s%3d %.2d:%.2d:%.2d %d\n", wday_name[timeptr->tm_wday], mon_name[timeptr->tm_mon], timeptr->tm_mday, timeptr->tm_hour, timeptr->tm_min, timeptr->tm_sec, 1900 + timeptr->tm_year); return result; }
This function is supposed to output a character string of 26 positions at most, including the terminating zero. If we count the length indicated by the format directives we arrive at 25:
o 3 for the day of the week +
o 1 space +
o 3 for the month +
o 3 for the day in the month +
o 1 space +
o 8 for the hour in hh:mm:ss format +
o 1 space +
o 4 for the year +
o 1 newline.
It makes 25 characters, and taking into account the terminating zero, the calculation appears sufficient is not.
The sprintf()
call has undefined behavior if the value of timeptr->tm_year
is outside the range -2899, 8099 and consequently the year being represented falls outside the range -999, 9999.
The problem is that the format %d of the printf specification doesn't allow for a maximum size. When you write %.3d it means that at least 3 characters will be output, but it could be much more if, for instance, the input is bigger than 999. In that case, the buffer allocated for asctime is too small to contain the printf result, resulting in a buffer overflow.
The asctime()
function primarily exists for compatibility with older implementations. Also, The asctime()
function does not support localized date and time formats. To avoid these problems, applications should use strftime()
to generate strings from broken-down times.
The POSIX standard developers decided to mark the asctime()
function obsolescent even though they are in C99 because of the possibility of buffer overflow. C99 also provides the strftime()
function which can be used to avoid these problems.
Noncompliant Code Example
Avoid using the asctime()
function.
struct tm time; time.tm_sec = 60; /* Seconds [0,60] */ time.tm_min = 59; /* Minutes [0,59] */ time.tm_hour = 23; /* Hour [0,23] */ time.tm_mday = 31; /* Day of month [1,31] */ time.tm_mon = 0; /* Month of year [0,11] */ time.tm_year = 8100; /* Years since 1900 */ time.tm_wday = 0; /* Day of week [0,6] (Sunday =0) */ time.tm_yday = 365; /* Day of year [0,365] */ time.tm_isdst = -1; /* Daylight Savings flag */ char *time = asctime(&time);
Compliant Solution
Use the strftime()
function.
char *s=; size_t maxsize; const char *format; const struct tm *timeptr; size_t size = strftime(s, maxsize, format, timeptr);
Risk Assessment
On implementations that do not detect output string length overflow, it is possible to overflow the output buffers resulting in a vulnerability.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
STR30-C |
high |
high |
low |
P9 |
L2 |
Automated Detection
Unknown.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as STR30-CPP. Do not attempt to modify string literals.
References
[[ISO/IEC 9899:1999]] Section 7.23.3.1, "The asctime function" and Section 7.23.3.5, "The strftime function"
http://www.opengroup.org/onlinepubs/9699919799/functions/asctime.html