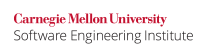
Alternative functions that limit the number of bytes copied are often recommended to mitigate buffer overflow vulnerabilities, for example:
strncpy()
instead ofstrcpy()
strncat()
instead ofstrcat()
fgets()
instead ofgets()
snprintf()
instead ofsprintf()
These function truncate strings that exceed the specified limits. Additionally, some functions such as strncpy()
do not guarantee that the resulting string is null-terminated [[STR33-C]].
Truncation results in a loss of data, and in some cases, leads to software vulnerabilities.
Non-Compliant Code Example
The standard function strncpy()
and strncat()
copy a specified number n
characters from a source string to a destination array. If there is no null character in the first n
characters of the source array the result is not be null-terminated and any remaining charactes are truncated
char *string_data; char a[16]; ... strncpy(a, string_data, sizeof(a));
Compliant Solution 1
Truncation resulting from a string copy operation should be treated as an error condition.
#define A_SIZE 16 char *string_data; char a[A_SIZE]; ... if (string_data) { if (strlen(string_data) < sizeof(a)) { strcpy(a, string_data); } else { /* handle string too large condition */ } } else { /* handle null string condition */ }
Compliant Solution 2
The strcpy_s()
function provides additional safeguards including accepting the size of the destination buffer as an additional argument [[STR00-A]].
#define A_SIZE 16 char *string_data; char a[A_SIZE]; ... if (string_data) { if (strlen(string_data) < sizeof(a)) { strcpy(a, sizeof(a), string_data); } else { /* handle string too large condition */ } } else { /* handle null string condition */ }
Exception
An exception to this rule applies if the intent of the programmer was to intentionally truncate the null-terminated byte string. To be compliant with this standard, this intent must be made clear statement in comments.
Priority: P2 Level: L1
Truncating strings can lead to a loss of data and exploitable vulnerabilities in some cases.
Component |
Value |
---|---|
Severity |
1 (medium) |
Likelihood |
1 (probable) |
Remediation cost |
2 (medium) |
References
- ISO/IEC 9899-1999 Section 7.21 String handling <string.h>
- Seacord 05a Chapter 2 Strings
- ISO/IEC TR 24731-2006