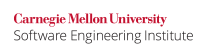
Files should be created with appropriate access permissions. Creating a file with insufficient file access permissions may allow unintended access to program-critical files.
Non-compliant Code Example 1
Using the POSIX function open()
to create a file but failing to provide access permissions for that file may cause that file to be created with unintended access permissions. This omission has been known to lead to vulnerabilities; for instance, CVE-2006-1174.
... int fd = open(file_name, O_CREAT | O_WRONLY); /* access permissions are missing */ if (fd == -1){ /* Handle Error */ } ...
Compliant Code Solution 1
Access permissions for the newly created file should be specified in the call to open()
.
... int fd = open(file_name, O_CREAT | O_WRONLY, file_access_permissions); if (fd == -1){ /* Handle Error */ } ...
Non-compliant Code Example 2
The fopen()
function does not provide a mechanism to specify file access permissions. In the example below, if the call to fopen()
creates a new file, the access permissions for that file will be implementation defined.
... FILE * fptr = fopen(file_name, "w"); if (!fptr){ /* Handle Error */ } ...
Compliant Code Solution 2
The fopen_s()
function defined in ISO/IEC TR 24731-2006 provides some control over file access permissions. Specifically, the report states: "If the file is being created, and the first character of the mode string is not 'u', to the extent that the underlying system supports it, the file shall have a file permission that prevents other users on the system from accessing the file."
... File *fptr; errno_t res = fopen_s(&fptr,file_name, "w"); if (res != 0) { /* Handle Error */ } ...
Risk Assessment
Creating files without appropriate access permissions may allow unintended access to those files.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO06-A |
2 (medium) |
1 (unlikely) |
2 (medium) |
P4 |
L3 |
References
- ISO/IEC 9899-1999 Section 7.19.5.3, The fopen function
- Open Group 04 The open function
- ISO/IEC TR 24731-2006 Section 6.5.2.1, The fopen_s function
- CVE-2006-1174