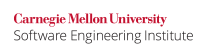
Referring to the value of errno
after a signal occurred other than as the result of calling the abort()
or raise()
function and the corresponding signal handler obtained a SIG_ERR
return from a call to the signal()
function.
Non-Compliant Code Example
If the request to register a signal handler can be honored, the signal()
function returns the value of the signal handler for the most recent successful call to the signal()
function for the specified signal. Otherwise, a value of
SIG_ERR
is returned and a positive value is stored in errno
.
#include <signal.h> #include <stdlib.h> #include <string.h> char *err_msg; volatile sig_atomic_t e_flag = 0; void handler(int signum) { signal(signum, handler); e_flag = 1; } int main(void) { signal(SIGINT, handler); err_msg = (char *)malloc(24); if (err_msg == NULL) { /* handle error condition */ } strcpy(err_msg, "No errors yet."); /* main code loop */ if (e_flag) { strcpy(err_msg, "SIGINT received."); } return 0; }
Compliant Solution
To be safe, signal handlers should only unconditionally set a flag of type volatile sig_atomic_t
and return.
#include <signal.h> #include <stdlib.h> #include <string.h> typedef void (*pfv)(int); char *err_msg; void handler(int signum) { pfv old_handler = signal(signum, handler); if (old_handler == SIG_ERR) { perror("SIGINT handler"); /* undefined behavior */ /* handle error condition */ } strcpy(err_msg, "SIGINT encountered."); } int main(void) { pfv old_handler = signal(SIGINT, handler); if (old_handler == SIG_ERR) { perror("SIGINT handler"); /* handle error condition */ } err_msg = (char *)malloc(24); if (err_msg == NULL) { /* handle error condition */ } strcpy(err_msg, "No errors yet."); /* main code loop */ return 0; }
Risk Assessment
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ERR32-C |
1 (low) |
1 (unlikely) |
3 (low) |
P3 |
L3 |
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 7.14.1.1, "The signal function"
ERR31-C. Don't redefine errno 13. Error Handling (ERR) ERR33-C. Only examine the value of errno when it is indicated to be valid by a function's return value