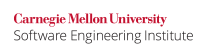
Perform file operations in a secure directory. In most cases, a secure directory is a directory no one other than the user, or possibly the administrator, has the ability to write, create, move, delete files or otherwise manipulate files. (Other users may read or search the directory, but generally may not modify the directory's contents in any way.)
Performing file operations in a secure directory eliminates the possibility that an attacker might tamper with the files or file system to exploit a file system vulnerability in a program. These vulnerabilities often exist because there is a loose binding between the file name and the actual file (see FIO01-A. Be careful using functions that use file names for identification). In some cases, file operations can be performed securely (and should be). In other cases, the only way to ensure secure file operations is to perform the operation within a secure directory.
Ensuring that file systems are configured in a safe manner is typically a system administration function. However, programs can often check that a file system is securely configured before performing file operations that may potentially lead to security vulnerabilities if the system is misconfigured. There is a slight possibility that file systems will be reconfigured in an insecure manner while a process is running and after the check has been made. As a result, it is always advisable to implement your code in a secure manner (that is, consistent with the other rules and recommendations in this section) even when running in a secure directory.
For examples on how to create a secure directory inside another secure directory see FIO15-A. Do not create temporary files in shared directories.
Non-Compliant Code Example
In this non-compliant code example, the file identified by file_name
is opened, processed, closed, and removed.
char *file_name; FILE *fp; /* initialize file_name */ fp = fopen(file_name, "w"); if (fp == NULL) { /* Handle error */ } /*... Process file ...*/ if (fclose(fp) != 0) { /* Handle error */ } if (remove(file_name) != 0) { /* Handle error */ }
An attacker can replace the file object identified by file_name
with a link to an arbitrary file before the call to fopen()
. It is also possible that the file object identified by file_name
in the call to remove()
is not the same file object identified by file_name
in the call to fopen()
. If the file is not in a secure directory, for example, /tmp/app/tmpdir/passwd, then an attacker can manipulate the location of the file as follows:
% cd /tmp/app/ % rm -rf tmpdir % ln -s /etc tmpdir
There is not much that can be programmatically done to ensure the file removed is the same file that was opened, processed, and closed except to make sure that the file is opened in a secure directory with privileges that would prevent the file from being manipulated by an untrusted user.
Compliant Solution (POSIX)
This example implementation of a secure_dir()
function will ensure that path
and all directories above it are owned either by the user or the superuser, and may not be modified by any other users. When checking directories, it is important to traverse from the root to the top in order to avoid a dangerous race condition where an attacker who has privileges to at least one of the directories can delete and recreate a directory after the privilege verification.
This function uses the realpath()
function to canonicalize the input path...see FIO02-A. Canonicalize path names originating from untrusted sources for more information on realpath()
. It then checks every directory in the canonical path, ensuring that every directory is owned by the current user or by root. Furthermore, every directory must disallow write access to everyone but the owner, either by turning off group write access and world write access, or by turning on the sticky bit.
#include <stdlib.h> #include <unistd.h> #include <limits.h> #include <libgen.h> #include <sys/stat.h> #include <string.h> /* Returns nonzero if directory is secure, zero otherwise */ int secure_dir(const char* path) { char *fullpath = NULL; char *path_copy = NULL; char *dirname_res = NULL; char ** dirs = NULL; int num_of_dirs = 0; int secure = 1; int i; struct stat buf; uid_t my_uid = geteuid(); /* * Canonicalize path and store the result in fullpath * see FIO02-A. Canonicalize path names originating from * untrusted sources */ if (!(path_copy = strdup(fullpath))) { /* Handle error */ } dirname_res = path_copy; /* Figure out how far it is to the root */ while (1) { dirname_res = dirname(dirname_res); num_of_dirs++; if ((strcmp(dirname_res, ".") == 0) || (strcmp(dirname_res, "/") == 0)) { break; } } free(path_copy); path_copy = NULL; /* Now allocate and fill the dirs array */ if (!(dirs = (char **)malloc(num_of_dirs*sizeof(*dirs)))) { /* Handle error */ } if (!(dirs[num_of_dirs - 1] = strdup(fullpath))) { /* Handle error */ } if(!(path_copy = strdup(fullpath))) { /* Handle error */ } dirname_res = path_copy; for (i = 1; i < num_of_dirs; i++) { dirname_res = dirname(dirname_res); dirs[num_of_dirs - i - 1] = strdup(dirname_res); } free(path_copy); path_copy = NULL; /* Traverse from the root to the top, checking * permissions along the way */ for (i = 0; i < num_of_dirs; i++) { if (stat(dirs[i], &buf) != 0) { /* Handle error */ } if ((buf.st_uid != my_uid) && (buf.st_uid != 0)) { /* Directory is owned by someone besides user or root */ secure = 0; } else if (!(buf.st_mode & S_ISVTX) && (buf.st_mode & (S_IWGRP | S_IWOTH))) { /* Others have permission to rename or remove files here */ secure = 0; } free(dirs[i]); dirs[i] = NULL; } free(dirs); dirs = NULL; return secure; }
This compliant solution uses the secure_dir()
function above to ensure that an attacker may not tamper with the file to be opened and subsequently removed.
char *file_name; FILE *fp; /* initialize file_name */ if (!secure_dir(file_name)) { /* Handle error */ } fp = fopen(file_name, "w"); if (fp == NULL) { /* Handle error */ } /*... Process file ...*/ if (fclose(fp) != 0) { /* Handle error */ } if (remove(file_name) != 0) { /* Handle error */ }
Risk Assessment
Failing to ensure proper permissions in a directory may lead to sensitive data getting saved to (or critical configuration or other input files being read from) public directories to which an attacker has access.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO15-A |
medium |
probable |
high |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]]
[[Open Group 04]] dirname()
,
realpath()
[[Viega 03]] Section 2.4, "Determining Whether a Directory Is Secure"
FIO16-A. Limit access to files by creating a jail 09. Input Output (FIO)