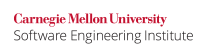
Alternative functions that limit the number of bytes copied are often recommended to mitigate buffer overflow vulnerabilities. For example
strncpy()
instead ofstrcpy()
strncat()
instead ofstrcat()
fgets()
instead ofgets()
snprintf()
instead ofsprintf()
These functions truncate strings that exceed the specified limits. Additionally, some functions such as strncpy()
do not guarantee that the resulting string is null-terminated (see STR32-C. Null-terminate byte strings as required).
Unintentional truncation results in a loss of data and, in some cases, leads to software vulnerabilities.
Non-Compliant Code Example
The standard functions strncpy()
and strncat()
copy a specified number of characters n
from a source string to a destination array. If there is no null character in the first n
characters of the source array, the result will not be null-terminated and any remaining characters are truncated.
char *string_data; char a[16]; /* ... */ strncpy(a, string_data, sizeof(a));
Compliant Solution (adequate space)
Either the strcpy()
or strncpy()
function can be used to copy a string and a null character to a destination buffer, provided there is enough space. Care must be taken to ensure that the destination buffer is large enough to hold the string to be copied and the NULL byte to prevent errors such as data truncation and buffer overflow.
char *string_data; char a[16]; if (string_data == NULL) { /* Handle null pointer error */ } else if (strlen(string_data) >= sizeof(a)) { /* Handle overlong string error */ } else { strcpy(a, string_data); }
It is assumed that string_data
is NULL terminated, that is, a NULL byte can be found within the bounds of the referenced character array. Otherwise, strlen()
will stray into other objects before finding a NULL byte.
Compliant Solution (TR 24731-1)
The strcpy_s()
function defined in [[ISO/IEC TR 24731-1:2007]] provides additional safeguards, including accepting the size of the destination buffer as an additional argument (see [STR07-A. Use TR 24731 for remediation of existing string manipulation code]). Also, strnlen_s()
accepts a maximum-length argument for strings that may not be null-terminated.
char *string_data; char a[16]; if (string_data == NULL) { /* Handle null pointer error */ } else if (strnlen_s(string_data, sizeof(a)) >= sizeof(a)) { /* Handle overlong string error */ } else { strcpy_s(a, sizeof(a), string_data); }
If a runtime constraint error is detected by either the call to strnlen_s()
or strcpy_s()
, the currently registered runtime-constraint handler is invoked. See ERR03-A. Use runtime-constraint handlers when calling functions defined by TR24731-1 for more information on using runtime-constraint handlers with TR 24731-1 functions.
Exceptions
STR03-EX1: The intent of the programmer is to intentionally truncate the null-terminated byte string.
Risk Assessment
Truncating strings can lead to a loss of data.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
STR03-A |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
The LDRA tool suite V 7.6.0 is able to detect violations of this recommendation.
Fortify SCA Version 5.0 with CERT C Rule Pack can detect violations of this recommendation.
The tool Compass / ROSE could detect violations in the following manner: All calls to strncpy()
and the other functions should be follwed by an assignment of a terminating character to null-terminate the string.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]] Section 7.21, "String handling <string.h>"
[[Seacord 05a]] Chapter 2, "Strings"
[[ISO/IEC TR 24731-1:2007]]
STR02-A. Sanitize data passed to complex subsystems 07. Characters and Strings (STR) STR04-A. Use plain char for characters in the basic character set