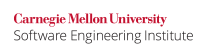
Creating a file with overly permissive access permissions may allow an unprivileged user to access that file. Although access permissions are heavily dependent on the file system, many file-creation functions provide mechanisms to set (or at least influence) access permissions. When these functions are used to create files, appropriate access permissions should be specified to prevent unintended access.
When setting access permissions, it is important to make sure that an attacker is not able to alter them (see FIO15-C. Ensure that file operations are performed in a secure directory).
Noncompliant Code Example: fopen()
The fopen()
function does not allow the programmer to explicitly specify file access permissions. In this noncompliant code example, if the call to fopen()
creates a new file, the access permissions are implementation-defined.
char *file_name; FILE *fp; /* initialize file_name */ fp = fopen(file_name, "w"); if (!fp){ /* handle Error */ }
Implementation Details
On POSIX-compliant systems, the permissions may be restricted by the value of the POSIX umask()
function [[Open Group 04]].
The operating system modifies the access permissions by computing the intersection of the inverse of the umask and the permissions requested by the process [[Viega 03]]. For example, if the variable requested_permissions
contained the permissions passed to the operating system to create a new file, the variable actual_permissions
would be the actual permissions that the operating system would use to create the file:
requested_permissions = 0666; actual_permissions = requested_permissions & ~umask();
For OpenBSD and Linux operating systems, any created files will have mode S_IRUSR|S_IWUSR|S_IRGRP|S_IWGRP|S_IROTH|S_IWOTH
(0666), as modified by the process's umask value. (See fopen(3)
in the OpenBSD Manual Pages [[OpenBSD]].)
Compliant Solution: fopen_s()
(ISO/IEC TR 24731-1)
The ISO/IEC TR 24731-1 function fopen_s()
can be used to create a file with restricted permissions [[ISO/IEC TR 24731-1:2007]]
If the file is being created, and the first character of the mode string is not 'u', to the extent that the underlying system supports it, the file shall have a file permission that prevents other users on the system from accessing the file. If the file is being created and the first character of the mode string is 'u', then by the time the file has been closed, it shall have the system default file access permissions.
The 'u' character can be thought of as standing for "umask," meaning that these are the same permissions that the file would have been created with had it been created by fopen()
.
char *file_name; FILE *fp; /* initialize file_name */ errno_t res = fopen_s(&fp, file_name, "w"); if (res != 0) { /* handle Error */ }
Noncompliant Code Example: open()
(POSIX)
Using the POSIX function open()
to create a file, but failing to provide access permissions for that file, may cause the file to be created with overly permissive access permissions. This omission has been known to lead to vulnerabilities (for instance, CVE-2006-1174).
char *file_name; int fd; /* initialize file_name */ fd = open(file_name, O_CREAT | O_WRONLY); /* access permissions were missing */ if (fd == -1){ /* handle Error */ }
This example also violates EXP37-C. Call functions with the arguments intended by the API.
Compliant Solution: open()
(POSIX)
Access permissions for the newly created file should be specified in the third argument to open()
. Again, the permissions are modified by the value of umask()
.
char *file_name; int file_access_permissions; /* initialize file_name and file_access_permissions */ int fd = open( file_name, O_CREAT | O_WRONLY, file_access_permissions ); if (fd == -1){ /* handle Error */ }
John Viega and Matt Messier also provide the following advice [[Viega 03]]:
Do not rely on setting the umask to a "secure" value once at the beginning of the program and then calling all file or directory creation functions with overly permissive file modes. Explicitly set the mode of the file at the point of creation. There are two reasons to do this. First, it makes the code clear; your intent concerning permissions is obvious. Second, if an attacker managed to somehow reset the umask between your adjustment of the umask and any of your file creation calls, you could potentially create sensitive files with wide-open permissions.
Risk Assessment
Creating files with weak access permissions may allow unintended access to those files.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO06-C |
medium |
probable |
high |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[CVE]]
[[ISO/IEC 9899:1999]] Section 7.19.5.3, "The fopen
function"
[[ISO/IEC PDTR 24772]] "XZN Missing or Inconsistent Access Control"
[[OpenBSD]]
[[Open Group 04]] "The open
function," "The umask
function"
[[ISO/IEC TR 24731-1:2007]] Section 6.5.2.1, "The fopen_s
function"
[[Viega 03]] Section 2.7, "Restricting Access Permissions for New Files on UNIX"
[[Dowd 06]] Chapter 9, "UNIX 1: Privileges and Files"