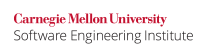
Sometimes, when a variable is declared final
, it is believed to be immutable. If the variable is a primitive type, declaring it final
means that its value cannot be subsequently changed. However, if the variable is a reference to a mutable object, the object's contained data that appears to be immutable, may actually be mutable. For example, a final
method parameter that is a reference to an object does not imply immutability of the object itself. The argument to this method uses pass-by-value to copy the reference but the referenced data remains mutable.
According to the Java Language Specification [[JLS 05]], section 4.12.4 "final
Variables":
... if a
final
variable holds a reference to an array, then the components of the array may be changed by operations on the array, but the variable will always refer to the same array.
Noncompliant Code Example
In this noncompliant code example, the values of instance fields a
and b
can be changed even after their initialization. When an object reference is declared final
, it only signifies that the reference cannot be changed, whereas the referenced contents can still be altered.
class FinalClass{ private int a; private int b; FinalClass(int a, int b){ this.a = a; this.b = b; } void set_ab(int a, int b){ this.a = a; this.b = b; } void print_ab(){ System.out.println("the value a is: " + this.a); System.out.println("the value b is: " + this.b); } } public class FinalCaller { public static void main(String[] args) { final FinalClass fc = new FinalClass(1, 2); fc.print_ab(); // change the value of a,b. fc.set_ab(5, 6); fc.print_ab(); } }
Compliant Solution
If a
and b
have to be kept immutable after their initialization, the simplest approach is to declare them as final
. However, this requires the elimination of the setter method set_ab()
.
private final int a; private final int b;
Compliant Solution
This compliant solution provides a clone()
method in the class and does not require the elimination of the setter method. The clone()
method returns a copy of the original object. This new object can be freely used without affecting the original object. Using the clone()
method allows the class to remain mutable. (OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely)
final public class NewFinal implements Cloneable { private int a; private int b; NewFinal(int a, int b){ this.a = a; this.b = b; } void print_ab(){ System.out.println("the value a is: "+ this.a); System.out.println("the value b is: "+ this.b); } void set_ab(int a, int b){ this.a = a; this.b = b; } public NewFinal clone() throws CloneNotSupportedException{ NewFinal cloned = (NewFinal) super.clone(); return cloned; } } public class NewFinalCaller { public static void main(String[] args) throws CloneNotSupportedException { final NewFinal nf = new NewFinal(1, 2); nf.print_ab(); // Get the copy of original object NewFinal nf2 = nf.clone(); // Change the value of a,b of the copy. nf2.set_ab(5, 6); // Original value will not be changed nf.print_ab(); } }
The class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be accessed and used, while preventing the fields from being modified, and complies with OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely.
Noncompliant Code Example
Another common mistake is to use a public static final
array. Clients can trivially modify the contents of the array (although they are unable to change the array itself, as it is final
). In this noncompliant code example, the elements of the items[]
array, are modifiable.
public static final String[] items = { ... };
Compliant Solution
This compliant solution defines a private
array and a public
method that returns a copy of the array.
private static final String[] items = { ... }; public static final String[] somethings() { return items.clone(); }
As a result, the original array values cannot be modified by a client.
Compliant Solution
This compliant solution declares a private
array from which a public
immutable list is constructed.
private static final String[] items = { ... }; public static final List<String> itemsList = Collections.unmodifiableList(Arrays.asList(items));
Neither the original array values nor the public
list can be modified by a client. For more details about unmodifiable wrappers, refer to SEC14-J. Provide sensitive mutable classes with unmodifiable wrappers.
Risk Assessment
Using final
to declare the reference to a mutable object is potentially misleading because the contents of the object can still be changed.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ03- J |
low |
probable |
medium |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Sections 4.12.4 "final Variables" and 6.6, "Access Control"
[[Bloch 08]] Item 13: Minimize the accessibility of classes and members
[[Core Java 04]] Chapter 6
[[MITRE 09]] CWE ID 607 "Public Static Final Field References Mutable Object"
OBJ08-J. Avoid using finalizers 08. Object Orientation (OBJ) OBJ04-J. Encapsulate the absence of an object by using a Null Object