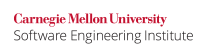
Programmers sometimes incorrectly believe that declaring a field or variable final
makes the referenced object immutable. Declaring variables that have a primitive type to be final
indeed prevents changes to their values after initialization (other than through the use of the unsupported sun.misc.Unsafe
class). When the variable has a reference type, however, the presence or absence of a final
clause in the declaration makes the reference itself immutable; the final
clause lacks any effect whatsoever on the referenced object. Consequently, the fields of the referenced object could, in fact, be mutable. Similarly, a final
method parameter obtains an immutable copy of the object reference; once again, this lacks any effect on the mutability of the referenced data.
According to the Java Language Specification [[JLS 2005]], Section 4.12.4, "
final
Variables"
... if a
final
variable holds a reference to an array, then the components of the array may be changed by operations on the array, but the variable will always refer to the same array.
Noncompliant Code Example (Mutable Class, final
Reference)
In this noncompliant code example, the values of instance fields x
and y
can be changed even after their initialization, even though the reference to the point
instance is declared to be final
.
class Point { private int x; private int y; Point(int x, int y){ this.x = x; this.y = y; } void set_xy(int x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } } public class PointCaller { public static void main(String[] args) { final Point point = new Point(1, 2); point.print_xy(); // change the value of x, y point.set_xy(5, 6); point.print_xy(); } }
When an object reference is declared final
, it signifies only that the reference cannot be changed; the mutability of the referenced contents remains unaffected.
Compliant Solution (final
Fields)
When the values of the x
and y
members must remain immutable after their initialization, they should be declared final
. However, this requires the elimination of the setter method set_xy()
.
class Point { private final int x; private final int y; Point(int x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } }
Compliant Solution (Provide Copy Functionality)
This compliant solution provides a clone()
method in the final
class Point
and does not require the elimination of the setter method.
final public class Point implements Cloneable { private int x; private int y; Point(int x, int y){ this.x = x; this.y = y; } void set_xy(int x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: "+ this.x); System.out.println("the value y is: "+ this.y); } public Point clone() throws CloneNotSupportedException{ Point cloned = (Point) super.clone(); // No need to clone x and y as they are primitives return cloned; } } public class PointCaller { public static void main(String[] args) throws CloneNotSupportedException { final Point point = new Point(1, 2); point.print_xy(); // Get the copy of original object Point pointCopy = point.clone(); // Change the value of x,y of the copy. pointCopy.set_xy(5, 6); // Original value remains unchanged point.print_xy(); } }
The clone()
method returns a copy of the original object and reflects its state at the moment of cloning . This new object can be freely used without exposing the original object. Using the clone()
method allows the class to remain mutable. (See guideline OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely.)
The Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object. This compliant solution complies with guideline OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely.
Noncompliant Code Example (Arrays)
This noncompliant code example uses a public static final
array, items
.
public static final String[] items = { ... };
Clients can trivially modify the contents of the array, even though declaring the array reference to be final
prevents modification of the reference itself.
Compliant Solution (Clone the Array)
This compliant solution defines a private
array and a public
method that returns a copy of the array.
private static final String[] items = { ... }; public static final String[] somethings() { return items.clone(); }
Consequently, the original array values cannot be modified by a client. Note that a manual deep copy could be required when dealing with arrays of objects. This generally happens when the objects do not export a clone()
method. Refer to guideline FIO00-J. Defensively copy mutable inputs and mutable internal components for more information.
Compliant Solution (Unmodifiable Wrappers)
This compliant solution declares a private
array from which a public
immutable list is constructed.
private static final String[] items = { ... }; public static final List<String> itemsList = Collections.unmodifiableList(Arrays.asList(items));
Neither the original array values nor the public
list can be modified by a client. For more details about unmodifiable wrappers, refer to guideline SEC14-J. Provide sensitive mutable classes with unmodifiable wrappers.
Risk Assessment
Using final
references to mutable objects can mislead unwary programmers because the contents of the referenced object remain mutable.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ01-J |
low |
probable |
medium |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
MITRE CWE: CWE-607 "Public Static Final Field References Mutable Object"
Bibliography
[[Bloch 2008]] Item 13: Minimize the accessibility of classes and members
[[Core Java 2004]] Chapter 6
[[JLS 2005]] Sections 4.12.4 "final Variables" and 6.6 "Access Control"
OBJ00-J. Declare data members as private and provide accessible wrapper methods 08. Object Orientation (OBJ) OBJ02-J. Do not ignore return values of methods that operate on immutable objects