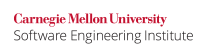
Immutability helps to supporting security reasoning. It is safe to share immutable objects, without risk that the recipient can modify something that we are relying upon [[Mettler 2010B]].
Programmers could incorrectly expect that declaring a field or variable final
makes the referenced object immutable. Declaring variables that have a primitive type to be final
does prevent changes to their values after initialization (unless the unsupported sun.misc.Unsafe
class is used). However, when the variable has a reference type, the presence of a final
clause in the declaration only makes the reference itself immutable. The final
clause has no effect on the referenced object. Consequently, the fields of the referenced object can be mutable. For example, according to the Java Language Specification [[JLS 2005]], §4.12.4, "
final
Variables,"
... if a
final
variable holds a reference to an array, then the components of the array may be changed by operations on the array, but the variable will always refer to the same array.
Similarly, a final
method parameter obtains an immutable copy of the object reference. Again, this has no effect on the mutability of the referenced data.
Noncompliant Code Example (Mutable Class, final
Reference)
In this noncompliant code example, the programmer has declared the reference to the point
instance to be final
, under the incorrect assumption that this prevents modification of the values of the instance fields x
and y
. The values of the instance fields can be changed after their initialization because the final
clause applies only to the reference to the point
instance and not the referenced object.
class Point { private int x; private int y; Point(int x, int y) { this.x = x; this.y = y; } void set_xy(int x, int y) { this.x = x; this.y = y; } void print_xy() { System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } } public class PointCaller { public static void main(String[] args) { final Point point = new Point(1, 2); point.print_xy(); // change the value of x, y point.set_xy(5, 6); point.print_xy(); } }
When an object reference is declared final
, it signifies only that the reference cannot be changed; the mutability of the referenced object is unaffected.
Compliant Solution (final
Fields)
When the values of the x
and y
members must remain immutable after their initialization, they should be declared final
. However, this invalidates a set_xy()
method because it can no longer change the values of x
and y
.
class Point { private final int x; private final int y; Point(int x, int y) { this.x = x; this.y = y; } void print_xy() { System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } // set_xy(int x, int y) no longer possible }
With this modification, the values of the instance fields become immutable and, consequently, match the programmer's intended usage model.
Compliant Solution (Provide Copy Functionality)
If the class must remain mutable, another compliant solution is to provide copy functionality. This compliant solution provides a clone()
method in the final
class Point
, avoiding the elimination of the setter method.
final public class Point implements Cloneable { private int x; private int y; Point(int x, int y) { this.x = x; this.y = y; } void set_xy(int x, int y) { this.x = x; this.y = y; } void print_xy() { System.out.println("the value x is: "+ this.x); System.out.println("the value y is: "+ this.y); } public Point clone() throws CloneNotSupportedException{ Point cloned = (Point) super.clone(); // No need to clone x and y as they are primitives return cloned; } } public class PointCaller { public static void main(String[] args) throws CloneNotSupportedException { final Point point = new Point(1, 2); point.print_xy(); // Get the copy of original object Point pointCopy = point.clone(); // pointCopy now holds a unique reference to the newly cloned Point instance // Change the value of x,y of the copy. pointCopy.set_xy(5, 6); // Original value remains unchanged point.print_xy(); } }
The clone()
method returns a copy of the original object that reflects the state of the original object at the moment of cloning. This new object can be used without exposing the original object. Because the caller holds the only reference to the newly cloned instance, the instance fields cannot be changed without the caller's cooperation. This use of the clone()
method allows the class to remain securely mutable. (See rule "OBJ04-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely.")
The Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object. This solution also complies with rule "OBJ04-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely."
Noncompliant Code Example (Arrays)
This noncompliant code example uses a public static final
array, items
.
public static final String[] items = {/* ... */};
Clients can trivially modify the contents of the array, even though declaring the array reference to be final
prevents modification of the reference itself.
Compliant Solution (Index Getter)
This compliant solution makes the array private
and provides public methods to get individual items and array size. Providing direct access to the array objects themselves is safe because String
is immutable.
private static final String[] items = {/* ... */}; public static final String getItem(int index) { return items[index]; } public static final int getItemCount() { return items.length; }
Compliant Solution (Clone the Array)
This compliant solution defines a private
array and a public
method that returns a copy of the array.
private static final String[] items = {/* ... */}; public static final String[] getItems() { return items.clone(); }
As a result, the original array values cannot be modified by a client. Note that a manual deep copy could be required when dealing with arrays of objects. This generally happens when the objects do not export a clone()
method. Refer to rule "OBJ06-J. Defensively copy mutable inputs and mutable internal components" for more information.
As before, this method provides direct access to the array objects themselves, which is safe because String
is immutable. If the array contained mutable objects, the getItems()
method could return a cloned array of cloned objects.
Compliant Solution (Unmodifiable Wrappers)
This compliant solution declares a private
array from which a public
immutable list is constructed.
private static final String[] items = { ... }; public static final List<String> itemsList = Collections.unmodifiableList(Arrays.asList(items));
Neither the original array values nor the public
list can be modified by a client. For more details about unmodifiable wrappers, refer to rule "void SEC14-J. Provide sensitive mutable classes with unmodifiable wrappers." This solution still applies if the array contains mutable items instead of String
.
Risk Assessment
Incorrectly assuming that final
references cause the contents of the referenced object to remain mutable can result in an attacker modifying an object thought by the programmer to be immutable.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ02-J |
low |
probable |
medium |
P4 |
L3 |
Related Guidelines
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="327ea872-a56d-4214-821d-3383fec76b4b"><ac:plain-text-body><![CDATA[ |
[[Bloch 2008 |
AA. Bibliography#Bloch 08]] |
Item 13: Minimize the accessibility of classes and members |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="b120bbf2-189b-47ef-88e2-af6daac3eaff"><ac:plain-text-body><![CDATA[ |
[[Core Java 2004 |
AA. Bibliography#Core Java 04]] |
Chapter 6 |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="7d5f32dd-37f8-49db-81dc-2cb652aa058c"><ac:plain-text-body><![CDATA[ |
[[JLS 2005 |
AA. Bibliography#JLS 05]] |
[§4.12.4 "final Variables" |
http://java.sun.com/docs/books /jls/third_edition/html/typesValues.html#4.12.4] ]]></ac:plain-text-body></ac:structured-macro> |
|
||||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="3af77c49-245c-4814-b1c3-2d609888c9b3"><ac:plain-text-body><![CDATA[ |
[[Mettler 2010B |
AA. Bibliography#Mettler 2010B]] |
|
]]></ac:plain-text-body></ac:structured-macro> |
OBJ02-J. Minimize the accessibility of classes and their members 04. Object Orientation (OBJ) OBJ04-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely