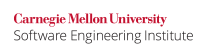
The WebView
class displays web pages as part of an activity layout. The behavior of a WebView
object can be customized using the WebSettings
object, which can be obtained from WebView.getSettings()
.
Major security concerns for WebView
are about the setJavaScriptEnabled()
, setPluginState()
, and setAllowFileAccess()
methods.
setJavaScriptEnabled()
The setJavaScriptEnabled()
tells WebView to enable JavaScript execution. To set it true:
webview.getWebSettings().setJavaScriptEnabled(true);
The default is false
.
setPluginState()
The setPluginState()
method tells the WebView to enable, disable, or have plugins enabled on demand.
ON | any object will be loaded even if a plugin does not exist to handle the content |
ON_DEMAND | if there is a plugin that can handle the content, a placeholder is shown until the user clicks on the placeholder. Once clicked, the plugin will be enabled on the page. |
OFF | all plugins will be turned off and any fallback content will be used |
The default is OFF
.
setAllowFileAccess()
The setAllowFileAccess()
method enables or disables file access within WebView.
The default is true
.
setAllowContentAccess()
The setAllowContentAccess()
method enables or disables content URL access within WebView. Content URL access allows WebView to load content from a content provider installed in the system.
The default is true
.
setAllowFileAccessFromFileURLs()
Sets whether JavaScript running in the context of a file scheme URL should be allowed to access content from other file scheme URLs. To enable the most restrictive, and therefore secure policy, this setting should be disabled. Note that the value of this setting is ignored if the value of getAllowUniversalAccessFromFileURLs()
is true
.
The default value is true
for API level ICE_CREAM_SANDWICH_MR1
(API level 15) and below, and false
for API level JELLY_BEAN
(API level 16) and above.
setAllowUniversalAccessFromFileURLs()
Sets whether JavaScript running in the context of a file scheme URL should be allowed to access content from any origin. This includes acess to content from other file scheme URLs. To enable the most restrictive, and therefore secure policy, this setting should be disabled.
The default value is true
for API level ICE_CREAM_SANDWICH_MR1
(API level 15) and below, and false
for API level JELLY_BEAN
(API level 16) and above.
Security Concerns for WebView
Class
When an activity has WebView
embedded to display web pages, any application can create and send an Intent
object with a given URI to the activity to request that a web page be displayed.
WebView
can recognize a variety of schemes, including the file:
scheme. A malicious application may create and store a crafted content on its local storage area, make it accessible with MODE_WORLD_READABLE
permission, and send the URI (using the file:
scheme) of this content to a target activity. The target activity renders this content.
When the target activity (webView
object) sets JavaScript enabled, it can be abused to access the target application’s resources.
Android 4.1 provides additional methods to control file scheme access:
WebSettings#setAllowFileAccessFromFileURLs
WebSettings#setAllowUniversalAccessFromFileURLs
Noncompliant Code Example
The following noncompliant code example uses the WebView
component with JavaScript enabled and processes any URI passed through Intent
without any validation:
public class MyBrowser extends Activity { @override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); WebView webView = (WebView) findViewById(R.id.webview); // turn on javascript WebSettings settings = webView.getSettings(); settings.setJavaScriptEnabled(true); String url = getIntent().getStringExtra("URL"); webView.loadUrl(url); } }
Proof of Concept
This code shows how the vulnerability can be exploited:
// Malicious application prepares some crafted HTML file, // places it on a local storage, makes accessible from // other applications. The following code sends an // intent to a target application (jp.vulnerable.android.app) // to make it access and process the malicious HTML file. String pkg = "jp.vulnerable.android.app"; String cls = pkg + ".DummyLauncherActivity"; String uri = "file:///[crafted HTML file]"; Intent intent = new Intent(); intent.setClassName(pkg, cls); intent.putExtra("url", uri); this.startActivity(intent);
Compliant Solution
Any URI received via an intent
from outside a trust-boundary should be validated before rendering it with WebView
. For example, the following code checks a received URI and rejects the "file:
" scheme URI. More generally, it allows only URIs that start with "http". (Note that "https" starts with "http".)
public class MyBrowser extends Activity { @override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); WebView webView = (WebView) findViewById(R.id.webview); String url = getIntent().getStringExtra("url"); if (!url.startsWith("http")) { /* Note: "https".startsWith("http") == true */ url = "about:blank"; } webView.loadUrl(url); } }
Risk Assessment
Allowing WebView
to access sensitive resources may result in information leaks.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DRD02-J | medium | probable | high | P6 | L2 |
Automated Detection
Automatic detection is not feasible.
Related Vulnerabilities
- JVN#59652356 Cybozu KUNAI for Android vulnerable in the WebView class
- JVN#99813183 Galapagos Browser vulnerable in the WebView class
- JVN#79301570 Angel Browser vulnerable in the WebView class
- JVN#77393797 Cybozu Live for Android vulnerable in the WebView class
- JVN#03015214 KUNAI Browser for Remote Service beta vulnerable in the WebView class
- JVN#46088915 Yahoo! Browser vulnerable in the WebView class
Related Guidelines
Android Application Secure Design / Secure Coding Guidebook by JSSEC | 4.9 Using WebView |
Bibliography
4.9 Using WebView |