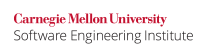
The calloc()
function takes two arguments: the number of elements to allocate and the storage size of those elements. Typically, calloc()
implementations multiply these arguments to determine how much memory to allocate. Historically, some implementations failed to check whether out-of-bounds results silently wrapped [RUS-CERT Advisory 2002-08:02]. If the result of multiplying the number of elements to allocate and the storage size wraps, less memory is allocated than was requested. As a result, it is necessary to ensure that these arguments, when multiplied, do not wrap.
Modern implementations of the C standard library should check for wrap. If the calloc()
function implemented by the libraries used for a particular implementation properly handles unsigned integer wrapping (in conformance with INT30-C. Ensure that unsigned integer operations do not wrap) when multiplying the number of elements to allocate and the storage size, that is sufficient to comply with this recommendation and no further action is required.
Noncompliant Code Example
In this noncompliant example, the user-defined function get_size()
(not shown) is used to calculate the size requirements for a dynamic array of long int
that is assigned to the variable num_elements
. When calloc()
is called to allocate the buffer, num_elements
is multiplied by sizeof(long)
to compute the overall size requirements. If the number of elements multiplied by the size cannot be represented as a size_t
, then calloc()
may allocate a buffer of insufficient size. When data is copied to that buffer, an overflow may occur.
size_t num_elements; long *buffer = (long *)calloc(num_elements, sizeof(long)); if (buffer == NULL) { /* Handle error condition */ } /* ... */ free(buffer); buffer = NULL;
Compliant Solution
In this compliant solution, the two arguments num_elements
and sizeof(long)
are checked before the call to calloc()
to determine if wrapping will occur:
long *buffer; size_t num_elements; if (num_elements > SIZE_MAX/sizeof(long)) { /* Handle error condition */ } buffer = (long *)calloc(num_elements, sizeof(long)); if (buffer == NULL) { /* Handle error condition */ }
Note that the maximum amount of allocatable memory is typically limited to a value less than SIZE_MAX
(the maximum value of size_t
). Always check the return value from a call to any memory allocation function in compliance with ERR33-C. Detect and handle standard library errors.
Risk Assessment
Unsigned integer wrapping in memory allocation functions can lead to buffer overflows that can be exploited by an attacker to execute arbitrary code with the permissions of the vulnerable process. Most implementations of calloc()
now check to make sure silent wrapping does not occur, but it is not always safe to assume the version of calloc()
being used is secure, particularly when using dynamically linked libraries.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM07-C | High | Unlikely | Medium | P6 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Astrée | 24.04 | Supported, but no explicit checker | |
CodeSonar | 8.1p0 | ALLOC.SIZE.MULOFLOW | Multiplication overflow of allocation size |
Compass/ROSE | |||
Parasoft C/C++test | 2023.1 | CERT_C-MEM07-a | The validity of values passed to library functions shall be checked |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
SEI CERT C++ Coding Standard | VOID MEM07-CPP. Ensure that the arguments to calloc(), when multiplied, can be represented as a size_t |
MITRE CWE | CWE-190, Integer overflow (wrap or wraparound) CWE-128, Wrap-around error |
Bibliography
[RUS-CERT] | Advisory 2002-08:02, "Flaw in calloc and Similar Routines" |
[Seacord 2013] | Chapter 4, "Dynamic Memory Management" |
[Secunia] | Advisory SA10635, "HP-UX calloc Buffer Size Miscalculation Vulnerability" |
10 Comments
Drew Yao
Is there an entry on this site regarding the similar overflow in the C++ new[] operator? I looked but couldn't find one. By the way, gcc still does not check if new[] overflows.
http://gcc.gnu.org/bugzilla/show_bug.cgi?id=19351
Microsoft's compiler does.
http://blogs.msdn.com/michael_howard/archive/2005/12/06/500629.aspx
Alex Volkovitsky
For the automatic detection, do we really expect everyone to have a size check before every call to
calloc()
even though it is only necessary on non C99 compliant systems? Seems like we'll be flagging a lot of code that no one will want to change....David Svoboda
I believe so, according to the letter of this rec. Probably this is a rec, not a rule, because following it strictly is probably unreasonable.
We can add exceptions to this rule to mitigate its 'unreasonability'. For instance, one could forego the check if the product is known at compile time.
Robert Seacord
This guideline became alot more reasonable with the inclusion of the "don't need to check if the library checks" clause.
Now I'm wondering if this shouldn't be promoted back to a rule?
David Svoboda
It prob is worth promoting (although we should prob clarify which standards require an explicit multiplciation check.)
This rule may be another in the set of 'rules for older C platforms, but not C11 (what about C99?)'. AFAIK EXP35-C is the only other rule in that set.
Robert Seacord
I don't think the langauge in the standard ever changed. I think this is just a matter of common implementation practice, which changed after the vulnerbility was publicized.
David Svoboda
Is it worthwhile for this rule to also handle multiplications inside malloc() or realloc()?
eg
Robert Seacord
This is not necessary, as it is covered by INT30-C. Ensure that unsigned integer operations do not wrap. This rule is here because the offense occurs "off stage", in a standard library function.
Yozo TODA
Can we rewrite this to the following?
"If the libraries ensure that all the code doing multiplication (including the code implementing calloc()) properly handle unsigned integer wrapping, that is sufficient to comply with this recommendation."
I think it means the library is compliant to "INT30-C. Ensure that unsigned integer operations do not wrap", hence an alternative phrase is
" If the library is compliant to INT30-C, then it means that integer wrapping does not happen inside calloc(). In this case, the library is compliant to this recommendation."
Robert Seacord
I made a change which may not have been exactly what you were asking for, but which might clarify further. In the narrow sense of this rule, it is only necessary for the a library to add one check for unsigned wrapping when multiplying the arguments provided to the calloc() function. If this check has been added, the application programmer can ignore this recommendation safely (even if wrap around checks elsewhere in the library have not been inserted).