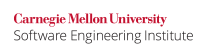
The definition of pointer arithmetic from the C++ Standard, [expr.add], paragraph 7 [ISO/IEC 14882-2014], states the following:
For addition or subtraction, if the expressions
P
orQ
have type “pointer to cvT
”, whereT
is different from the cv-unqualified array element type, the behavior is undefined. [Note: In particular, a pointer to a base class cannot be used for pointer arithmetic when the array contains objects of a derived class type. —end note]
Pointer arithmetic does not account for polymorphic object sizes, and attempting to perform pointer arithmetic on a polymorphic object value results in undefined behavior.
The C++ Standard, [expr.sub], paragraph 1 [ISO/IEC 14882-2014], defines array subscripting as being identical to pointer arithmetic. Specifically, it states the following:
The expression
E1[E2]
is identical (by definition) to*((E1)+(E2))
.
Do not use pointer arithmetic, including array subscripting, on polymorphic objects.
The following code examples assume the following static variables and class definitions.
int globI; double globD; struct S { int i; S() : i(globI++) {} }; struct T : S { double d; T() : S(), d(globD++) {} };
Noncompliant Code Example (Pointer Arithmetic)
In this noncompliant code example, f()
accepts an array of S
objects as its first parameter. However, main()
passes an array of T
objects as the first argument to f()
, which results in undefined behavior due to the pointer arithmetic used within the for
loop.
#include <iostream> // ... definitions for S, T, globI, globD ... void f(const S *someSes, std::size_t count) { for (const S *end = someSes + count; someSes != end; ++someSes) { std::cout << someSes->i << std::endl; } } int main() { T test[5]; f(test, 5); }
Noncompliant Code Example (Array Subscripting)
In this noncompliant code example, the for
loop uses array subscripting. Since array subscripts are computed using pointer arithmetic, this code also results in undefined behavior.
#include <iostream> // ... definitions for S, T, globI, globD ... void f(const S *someSes, std::size_t count) { for (std::size_t i = 0; i < count; ++i) { std::cout << someSes[i].i << std::endl; } } int main() { T test[5]; f(test, 5); }
Compliant Solution (Array)
Instead of having an array of objects, an array of pointers solves the problem of the objects being of different sizes, as in this compliant solution.
#include <iostream> // ... definitions for S, T, globI, globD ... void f(const S * const *someSes, std::size_t count) { for (const S * const *end = someSes + count; someSes != end; ++someSes) { std::cout << (*someSes)->i << std::endl; } } int main() { S *test[] = {new T, new T, new T, new T, new T}; f(test, 5); for (auto v : test) { delete v; } }
The elements in the arrays are no longer polymorphic objects (instead, they are pointers to polymorphic objects), and so there is no undefined behavior with the pointer arithmetic.
Compliant Solution (std::vector
)
Another approach is to use a standard template library (STL) container instead of an array and have f()
accept iterators as parameters, as in this compliant solution. However, because STL containers require homogeneous elements, pointers are still required within the container.
#include <iostream> #include <vector> // ... definitions for S, T, globI, globD ... template <typename Iter> void f(Iter i, Iter e) { for (; i != e; ++i) { std::cout << (*i)->i << std::endl; } } int main() { std::vector<S *> test{new T, new T, new T, new T, new T}; f(test.cbegin(), test.cend()); for (auto v : test) { delete v; } }
Risk Assessment
Using arrays polymorphically can result in memory corruption, which could lead to an attacker being able to execute arbitrary code.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CTR56-CPP | High | Likely | High | P9 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Axivion Bauhaus Suite | 7.2.0 | CertC++-CTR56 | |
CodeSonar | 8.1p0 | LANG.STRUCT.PARITH | Pointer Arithmetic |
Helix QAC | 2024.1 | C++3073 | |
Parasoft C/C++test | 2023.1 | CERT_CPP-CTR56-a | Don't treat arrays polymorphically |
LDRA tool suite | 9.7.1
| 567 S | Enhanced Enforcement |
Polyspace Bug Finder | R2023b | CERT C++: CTR56-CPP | Checks for pointer arithmetic on polymorphic object (rule fully covered) |
PVS-Studio | 7.30 | V777 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Bibliography
[ISO/IEC 14882-2014] | Subclause 5.7, "Additive Operators" |
[Lockheed Martin 2005] | AV Rule 96, "Arrays shall not be treated polymorphically" |
[Meyers 1996] | Item 3, "Never Treat Arrays Polymorphically" |
[Stroustrup 2006] | "What's Wrong with Arrays?" |
[Sutter 2004] | Item 100, "Don't Treat Arrays Polymorphically" |
2 Comments
Jussi Auvinen
In the code examples, the variables GlobI and GlobD are not initialized. This violates the EXP53-CPP, right?
Aaron Ballman
Because those variables are not local, they have static storage duration ([basic.std.static]p1) and all variables with static storage duration but no explicit initializer are zero-initialized ([basic.start.init]p2, [dcl.init]p6). So while they do not have an explicit initialization, they are still initialized to have the value
0
at program startup, which does not violate EXP53-CPP. There's a single sentence in that rule that talks about this: "Objects of static or thread storage duration are zero-initialized before any other initialization takes place [ISO/IEC 14882-2014] and need not be explicitly initialized before having their value read."