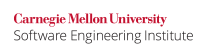
Unless coded properly, a while
or for
loop may execute forever or until the counter wraps around and reaches its final value. (See NUM00-J. Detect or prevent integer overflow.) This problem may result from incrementing or decrementing a loop counter by more than one and then testing for equality to a specified value to terminate the loop. In this case, it is possible that the loop counter will leapfrog the specified value and execute either forever or until the counter wraps around and reaches its final value. This problem may also be caused by naïve testing against limits—for example, looping while a counter is less than or equal to Integer.MAX_VALUE
or greater than or equal to Integer.MIN_VALUE
.
Noncompliant Code Example
This noncompliant code example appears to iterate five times:
for (i = 1; i != 10; i += 2) { // ... }
However, the loop never terminates. Successive values of i
are 1, 3, 5, 7, 9, 11, and so on; the comparison with 10 never evaluates to true
. The value reaches the maximum representable positive number (Integer.MAX_VALUE
), then wraps to the second-lowest negative number (Integer.MIN_VALUE + 1
). It then works its way up to –1, then 1, and proceeds as described earlier.
Noncompliant Code Example
This noncompliant code example terminates but performs more iterations than expected:
for (i = 1; i != 10; i += 5) { // ... }
Successive values of i
are 1, 6, and 11, skipping 10. The value of i
then wraps from near the maximum positive value to near the lowest negative value and works its way up toward 0. It then assumes 2, 7, and 12, skipping 10 again. After the value wraps from the high-positive to the low-negative side three more times, it finally reaches 0, 5, and 10, terminating the loop.
Compliant Solution
One solution is to simply ensure the loop termination condition is reached before the counter inadvertently wraps.
for (i = 1; i == 11; i += 2) { // ... }
This solution can be fragile when one or more of the conditions affecting the iteration must be changed. A better solution is to use a numerical comparison operator (that is, <
, <=
, >
, or >=
) to terminate the loop.
for (i = 1; i <= 10; i += 2) { // ... }
This latter solution can be more robust in the event of changes to the iteration conditions. However, this approach should never replace careful consideration regarding the intended and actual number of iterations.
Noncompliant Code Example
A loop expression that tests whether a counter is less than or equal to Integer.MAX_VALUE
or greater than or equal to Integer.MIN_VALUE
will never terminate because the expression will always evaluate to true
. For example, the following loop will never terminate because i
can never be greater than Integer.MAX_VALUE
:
for (i = 1; i <= Integer.MAX_VALUE; i++) { // ... }
Compliant Solution
The loop in this compliant solution terminates when i is equal to Integer.MAX_VALUE
:
for (i = 1; i != Integer.MAX_VALUE; i++) { // ... }
If the loop is meant to iterate for every value of i
greater than 0, including Integer.MAX_VALUE
, it can be implemented as follows:
i = 0; do { i++ // ... } while (i != Integer.MAX_VALUE);
Noncompliant Code Example
This noncompliant code example initializes the loop counter i
to 0 and then increments it by 2 on each iteration, basically enumerating all the even, positive values. The loop is expected to terminate when i
is greater than Integer.MAX_VALUE - 1
, an even value. In this case, the loop fails to terminate because the counter wraps around before becoming greater than Integer.MAX_VALUE - 1
.
for (i = 0; i <= Integer.MAX_VALUE - 1; i += 2) { // ... }
Compliant Solution
The loop in this compliant solution terminates when the counter i is greater than Integer.MAX_VALUE minus the step value as the loop-terminating condition.
for (i = 0; i <= Integer.MAX_VALUE - 2; i += 2) { // ... }
Applicability
Incorrect termination of loops may result in infinite loops, poor performance, incorrect results, and other problems. In any of the conditions used to terminate a loop can be influenced by an attacker, these errors can be exploited to cause a denial of service or other attack.
Automated Detection
Bibliography
7 Comments
James Ahlborn
i find the title of this rule confusing because I would consider the "!=" operator to be an "inequality" operator. is there some official definition of the term "inequality" that i'm missing?
Also, the handling of the MAX_VALUE is a nice recommendation, however i'm not sure it fits under the rule's current title.
David Svoboda
'inequality operator' refers to < <= > >= in mathematics.
!=
is always called 'not equal" not inequality.James Ahlborn
maybe so, but a google search for "inequality operator" turns up a lot of references to
!=
. since the target audience is programmers not mathematicians, maybe a better term should be used?Fred Long
James is correct. The JLS calls them "numerical comparison operators" (see the reference) so that is the term we should use. I've changed the title and text accordingly.
Dan C. Wlodarski
In the second "Noncompliant Code Example," the for loop's conditional is as follows:
i <= Integer.MAX_VALUE
However, that conditional is identical to the conditional in the for loop of the associated Compliant Code Example.
David Svoboda
Fixed, thanks
Dan C. Wlodarski
Thank you for the fix and the additional examples.