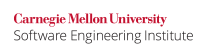
Integer variables are frequently intended to represent either a numeric value or a bit collection. Numeric values must be exclusively operated on using arithmetic operations, whereas bit collections must be exclusively operated on using bitwise operations. Bitwise operators include the unary operator ~ and the binary operators <<
, >>
, >>>
, &
, ^, and |
.
Performing bitwise and arithmetic operations on the same data can obscure the purpose of the data stored in the variable and often indicates confusion regarding that purpose. Unfortunately, bitwise operations are frequently performed on arithmetic values as a form of premature optimization. Although such operations are valid and will compile, they can reduce code readability.
Noncompliant Code Example (Left Shift)
Left- and right-shift operators are often employed to multiply or divide a number by a power of two. This approach compromises code readability and portability for the sake of often-illusory speed gains. The Java Virtual Machine (JVM) usually makes such optimizations automatically, and, unlike a programmer, the JVM can optimize for the implementation details of the current platform. This noncompliant code example includes both bitwise and arithmetic manipulations of the integer x
that conceptually contains a numeric value. The result is a prematurely optimized statement that assigns the value 5x + 1
to x
, which is what the programmer intended to express.
int x = 50; x += (x << 2) + 1;
Noncompliant Code Example (Left Shift)
This noncompliant code example segregates arithmetic and bitwise operators by variables. The x
variable participates only in bitwise operations, and y
participates only in arithmetic operations.
int x = 50; int y = x << 2; x += y + 1;
This example is noncompliant because the actual data has both bitwise and arithmetic operations performed on it, even though the operations are performed on different variables.
Compliant Solution (Left Shift)
In this compliant solution, the assignment statement is modified to reflect the arithmetic nature of x
, resulting in a clearer indication of the programmer's intentions.
int x = 50; x = 5 * x + 1;
A reviewer could now recognize that the operation should also be checked for overflow. The need for an overflow check might not have been apparent in the original, noncompliant code example (see NUM00-J. Detect or prevent integer overflow for more information).
Noncompliant Code Example (Logical Right Shift)
In this noncompliant code example, the programmer wishes to divide x
by 4. In a misguided attempt to optimize performance, the programmer uses a right-shift operation rather than a division operation.
int x = -50; x >>>= 2;
The >>>=
operator is a logical right shift; it fills the leftmost bits with zeroes, regardless of the number's original sign. After execution of this code sequence, x
contains a large positive number (specifically, 0x3FFFFFF3
). Using logical right shift for division produces an incorrect result when the dividend (x
in this example) contains a negative value.
Noncompliant Code Example (Arithmetic Right Shift)
In this noncompliant code example, the programmer attempts to correct the previous example by using an arithmetic right shift (the >>=
operator):
int x = -50; x >>= 2;
After this code sequence is run, x
contains the value -13
rather than the expected -12
. Arithmetic right shift truncates the resulting value toward negative infinity, whereas integer division truncates toward zero.
Compliant Solution (Right Shift)
In this compliant solution, the right shift is replaced by division.
int x = -50; x /= 4;
Noncompliant Code Example
In this noncompliant code example, a programmer attempts to fetch four values from a byte array and pack them into the integer variable result
. The integer value in this example represents a bit collection, not a numeric value.
// b[] is a byte array, initialized to 0xff byte[] b = new byte[] {-1, -1, -1, -1}; int result = 0; for (int i = 0; i < 4; i++) { result = ((result << 8) + b[i]); }
In the bitwise operation, the value of the byte array element b[i]
is promoted to an int
by sign extension. When a byte array element contains a negative value (for example, 0xff
), the sign extension propagates 1-bits into the upper 24 bits of the int
. This behavior might be unexpected if the programmer is assuming that byte
is an unsigned type. In this example, adding the promoted byte values to result
fails to result in a packed integer representation of the bytes [FindBugs 2008].
Noncompliant Code Example
This noncompliant code example masks off the upper 24 bits of the promoted byte array element before performing the addition. The number of bits required to mask the sizes of byte
and int
are specified by The Java Language Specification. Although this code calculates the correct result, it violates this rule by combining bitwise and arithmetic operations on the same data.
byte[] b = new byte[] {-1, -1, -1, -1}; int result = 0; for (int i = 0; i < 4; i++) { result = ((result << 8) + (b[i] & 0xff)); }
Compliant Solution
This compliant solution masks off the upper 24 bits of the promoted byte array element. The result is then combined with result
using a logical OR operation.
byte[] b = new byte[] {-1, -1, -1, -1}; int result = 0; for (int i = 0; i < 4; i++) { result = ((result << 8) | (b[i] & 0xff)); }
Exceptions
NUM01-EX0: Bitwise operations may be used to construct constant expressions.
int limit = (1 << 17) - 1; // 2^17 - 1 = 131071
Nevertheless, as a matter of style, it is preferable to replace such constant expressions with the equivalent hexadecimal constants.
int limit = 0x1FFFF; // 2^17 - 1 = 131071
NUM01-J-EX1: Data that is normally treated arithmetically may be treated with bitwise operations for the purpose of serialization or deserialization. This alternative treatment is often required for reading or writing the data from a file or network socket. Bitwise operations are also permitted when reading or writing the data from a tightly packed data structure of bytes.
int value = /* Interesting value */ Byte[] bytes = new Byte[4]; for (int i = 0; i < bytes.length; i++) { bytes[i] = value >> (i*8) & 0xFF; } /* bytes[] now has same bit representation as value */
Risk Assessment
Performing bitwise manipulation and arithmetic operations on the same variable obscures the programmer's intentions and reduces readability. Consequently, it is more difficult for a security auditor or maintainer to determine which checks must be performed to eliminate security flaws and ensure data integrity. For instance, overflow checks are critical for numeric types that undergo arithmetic operations but less critical for numeric types that undergo bitwise operations.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
NUM01-J | Medium | Unlikely | Medium | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Parasoft Jtest | 2023.1 | CERT.NUM01.BADSHIFT CERT.NUM01.NCBAV | Avoid incorrect shift operations Do not perform bitwise and arithmetic operations on the same data |
Related Guidelines
INT14-C. Avoid performing bitwise and arithmetic operations on the same data | |
VOID INT14-CPP. Avoid performing bitwise and arithmetic operations on the same data |
7 Comments
Yitzhak Mandelbaum
NCE (Logical Right Shift) and NCE (Arithmetic Right Shift) seem overly subjective. They contain no conflicting use of the variable x. Rather, we are just stating that x is intended for arithmetic use. I think we should limit the rule to the case of conflicting uses of the same variable. If not, then we should make explicit how we judge the "kind" of an integer variable in the absence of any external indications of its kind.
Yitzhak Mandelbaum
Is it permitted to compare two values of different kind? Seems like it should be forbidden, but I don't know the practical implications such a restriction.
David Svoboda
Good question. I'd say it is permitted, simply because no one has tried to argue otherwise.
Yitzhak Mandelbaum
I found this snippet in com.jcraft.jsch.ChannelX11, line 222:
in particular
+((-plen)&3)
.I'm not sure what to make of it -- should it be allowed or not? As far as I can tell, it is ensuring that the second argument is word aligned (for a 4 byte word). The method used is very direct, yet it seems that it should be an arithmetic calculation, not the bit trick used here. Still, i'm skeptical that this kind of optimization would be found by a compiler. The arithmetic version would be
4 - (plen % 4)
Dean Sutherland
I would expect any decent compiler to generate the same code for either expression. This kind of algebraic transformation is very important for decent performance; getting rid of div/mod/rem operations is a relatively big deal due to their high cost.
A Bishop
The second NC: "Noncompliant Code Example (Left Shift)"
shouldn't it be:
int x = 50;
int y = x << 2;
y += x + 1;
otherwise the code result is not the 5x +1 explained earlier but 8x +1.
David Svoboda
Yes it should. I've fixed it, thanks!