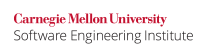
Division and remainder operations performed on integers are susceptible to divide-by-zero errors. Consequently, the divisor in a division or remainder operation on integer types must be checked for zero prior to the operation. Division and remainder operations performed on floating-point numbers are not subject to this rule.
Noncompliant Code Example (Division)
The result of the /
operator is the quotient from the division of the first arithmetic operand by the second arithmetic operand. Division operations are susceptible to divide-by-zero errors. Overflow can also occur during two's-complement signed integer division when the dividend is equal to the minimum (negative) value for the signed integer type and the divisor is equal to −1 (see NUM00-J. Detect or prevent integer overflow for more information). This noncompliant code example can result in a divide-by-zero error during the division of the signed operands num1
and num2
:
long num1, num2, result; /* Initialize num1 and num2 */ result = num1 / num2;
Compliant Solution (Division)
This compliant solution tests the divisor to guarantee there is no possibility of divide-by-zero errors:
long num1, num2, result; /* Initialize num1 and num2 */ if (num2 == 0) { // Handle error } else { result = num1 / num2; }
Noncompliant Code Example (Remainder)
The %
operator provides the remainder when two operands of integer type are divided. This noncompliant code example can result in a divide-by-zero error during the remainder operation on the signed operands num1
and num2
:
long num1, num2, result; /* Initialize num1 and num2 */ result = num1 % num2;
Compliant Solution (Remainder)
This compliant solution tests the divisor to guarantee there is no possibility of a divide-by-zero error:
long num1, num2, result; /* Initialize num1 and num2 */ if (num2 == 0) { // Handle error } else { result = num1 % num2; }
Risk Assessment
A division or remainder by zero can result in abnormal program termination and denial-of-service (DoS).
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
NUM02-J | Low | Likely | Medium | P6 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Coverity | 7.5 | DIVIDE_BY_ZERO | Implemented |
Parasoft Jtest | 2023.1 | CERT.NUM02.ZERO | Avoid division by zero |
PVS-Studio | 7.30 | V6020 | |
SonarQube | 9.9 | S3518 | Zero should not be a possible denominator |
Related Guidelines
INT33-C. Ensure that division and remainder operations do not result in divide-by-zero errors | |
CWE-369, Divide by Zero |
Bibliography
Subclause 6.5.5, "Multiplicative Operators" | |
Chapter 5, "Integers" | |
[Seacord 2015] | |
Chapter 2, "Basics" |
6 Comments
Dhruv Mohindra
David Svoboda
Fixed. For 2nd point, we have not been particularly consistent about how to handle errors...is there some normative text all code should rely on? My suggestion would be "// Handle Error" (except in cases where more specific directions are called for.)
Robert Seacord
I don't believe there is modulo operator in Java. There is a remainder operator.
http://docs.oracle.com/javase/specs/jls/se7/html/jls-15.html#jls-15.17.3
Julian Ospald
This seems to be covered in SonarQube too by S3518.
Jérôme GUY
We could specify the difference between integers and floatting point numbers.
David Svoboda
I clarified that this rule only applies to integers.