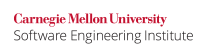
Immutability of fields prevents inadvertent modification as well as malicious tampering so that defensive copying while accepting input or returning values is unnecessary. However, some sensitive classes cannot be immutable. Fortunately, read-only access to mutable classes can be granted to untrusted code using unmodifiable wrappers. For example, the Collection
classes include a set of wrappers that allow clients to observe an unmodifiable view of a Collection
object.
Noncompliant Code Example
This noncompliant code example consists of class Mutable
, which allows the internal array object to be modified:
class Mutable { private int[] array = new int[10]; public int[] getArray() { return array; } public void setArray(int[] i) { array = i; } } // ... private Mutable mutable = new Mutable(); public Mutable getMutable() {return mutable;}
An untrusted invoker may call the mutator method setArray()
and violate the object's immutability property. Invoking the getter method getArray()
also allows modification of the private internal state of the class. This class also violates OBJ05-J. Do not return references to private mutable class members.
Noncompliant Code Example
This noncompliant code example extends the Mutable
class with a MutableProtector
subclass:
class MutableProtector extends Mutable { @Override public int[] getArray() { return super.getArray().clone(); } } // ... private Mutable mutable = new MutableProtector(); // May be safely invoked by untrusted caller having read ability public Mutable getMutable() {return mutable;}
In this class, invoking the getter method getArray()
does not allow modification of the private internal state of the class, in accordance with OBJ05-J. Do not return references to private mutable class members. However, an untrusted invoker may call the method setArray()
and modify the Mutable
object.
Compliant Solution
In general, sensitive classes can be transformed into safe-view objects by providing appropriate wrappers for all methods defined by the core interface, including the mutator methods. The wrappers for the mutator methods must throw an UnsupportedOperationException
so that clients cannot perform operations that affect the immutability property of the object.
This compliant solution adds a setArray()
method that overrides the Mutable.setArray()
method and prevents mutation of the Mutable
object:
class MutableProtector extends Mutable { @Override public int[] getArray() { return super.getArray().clone(); } @Override public void setArray(int[] i) { throw new UnsupportedOperationException(); } } // ... private Mutable mutable = new MutableProtector(); // May be safely invoked by untrusted caller having read ability public Mutable getMutable() {return mutable; }
The MutableProtector
wrapper class overrides the getArray()
method and clones the array. Although the calling code gets a copy of the mutable object's array, the original array remains unchanged and inaccessible. The overriding setArray()
method throws an exception if the caller attempts to use this method on the returned object. This object can be passed to untrusted code when read access to the data is permissible.
Applicability
Failure to provide an unmodifiable, safe view of a sensitive mutable object to untrusted code can lead to malicious tampering and corruption of the object.
Automated Detection
Tool | Version | Checker | Description |
---|
2 Comments
David Svoboda
I'm happy with this rule now. It may belong in the OBJ section...I don't like having rules in SEC that aren't about the security manager or permissions.
Fred Long
It's now in the OBJ section.