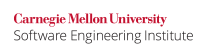
Java Coding Guidelines focuses on the Java SE 7 Platform environment and includes guidelines that address the issue of secure coding using the Java SE 7 API. The Java Language Specification: Java SE 7 Edition [JLS 2013] prescribes the behavior of the Java programming language and serves as the primary reference for the development of these guidelines.
Traditional languages standards, such as those for C and C++, include undefined, unspecified, and implementation-defined behaviors that can lead to vulnerabilities when a programmer makes incorrect assumptions about the portability of these behaviors. By contrast, the JLS more completely specifies language behaviors because Java is designed to be a cross-platform language. Even then, certain behaviors are left to the discretion of the implementer of the Java Virtual Machine (JVM) or the Java compiler. These guidelines identify such language peculiarities, suggest solutions to help implementers address the issues, and let programmers appreciate and understand the limitations of the language and navigate around them.
Focusing only on language issues does not translate to writing reliable and secure software. Design issues in Java APIs sometimes lead to their deprecation. At other times, the APIs or the relevant documentation may be interpreted incorrectly by the programming community. These guidelines identify such problematic APIs and highlight their correct use. Examples of commonly used faulty design patterns and idioms are also included.
The Java language, its core and extension APIs, and the JVM provide several security features, such as the security manager and access controller, cryptography, automatic memory management, strong type checking, and bytecode verification. These features provide sufficient security for most applications, but their proper use is of paramount importance. These guidelines highlight the pitfalls and caveats associated with the security architecture and stress its correct implementation. Adherence to these guidelines safeguards trusted programs from a plethora of exploitable security bugs that can cause denial of service, information leaks, erroneous computations, and privilege escalation.
Included Libraries
Figure 1.1 is a conceptual diagram of Oracle's Java SE products.
Figure 1.1 Java conceptual diagram of Oracle's Java SE products. (From Oracle Java SE Documentation. Copyright © 1995, 2010, Oracle and/or its affiliates. All rights reserved.)
These coding guidelines address security issues primarily applicable to the lang
and util
base libraries as well as for "other base libraries." They avoid the inclusion of open bugs that have already been marked to be fixed and those that lack negative ramifications. A functional bug is included only if it is likely to occur with high frequency, causes considerable security or reliability concerns, or affects most Java technologies that rely on the core platform. These guidelines are not limited to security issues specific to the core API but also include important reliability and security concerns pertaining to the standard extension APIs (javax
package).
Demonstrating the full range of security features that Java offers requires studying interaction of code with other components and frameworks. Occasionally, the coding guidelines use examples from popular web and application frameworks such as Spring and Struts and technologies such as Java Server Pages (JSP) to highlight a security vulnerability that cannot be examined in isolation. Only when the standard API provides no option to mitigate a vulnerability are third-party libraries and solutions suggested.
Issues Not Addressed
A number of issues are not addressed by this secure coding standard.
Content
These coding guidelines apply broadly to all platforms; concerns specific to only one Java-based platform are beyond the scope of these guidelines. For example, guidelines that are applicable to Android, Java Micro Edition (ME), or Java Enterprise Edition (EE) alone and not to Java Standard Edition (SE) are typically excluded. In Java SE, APIs that deal with the user interface (user interface toolkits) or the web interface for providing features such as sound, graphical rendering, user account access control, session management, authentication, and authorization are beyond the scope of these guidelines. Nevertheless, the guidelines discuss networked Java systems in light of the risks associated with improper input validation and injection flaws and suggest appropriate mitigation strategies. These guidelines assume that the functional specification of the product correctly identifies and prevents higher-level design and architectural vulnerabilities.
Coding Style
Coding style issues are subjective; it has proven impossible to develop a consensus on appropriate style guidelines. Consequently, Java™ Coding Guidelines generally avoids requiring enforcement of any particular coding style. Instead, we suggest that the user define style guidelines and apply those guidelines consistently. The easiest way to consistently apply a coding style is with the use of a code formatting tool. Many integrated development environments (IDEs) provide such capabilities.
Tools
Many of these guidelines are not amenable to automatic detection or correction. It some cases, tool vendors may choose to implement checkers to identify violations of these guidelines. As a federally funded research and development center (FFRDC), the Software Engineering Institute (SEI) is not in a position to recommend particular vendors or tools for this purpose.
Controversial Guidelines
In general, The CERT Oracle Java Coding Guidelines tries to avoid the inclusion of controversial guidelines that lack a broad consensus.