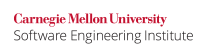
Code that is never executed is known as dead code. Typically, the presence of dead code indicates that a logic error has occurred as a result of changes to a program or the program's environment. To improve readability and ensure that logic errors are resolved, dead code should be identified, understood, and eliminated.
Noncompliant Code Example
This noncompliant code example contains code that cannot possibly execute.
sub fix_name { my $name = shift; if ($name eq "") { return $name; } $name =~ s/^([a-z])/\U$1\E/g; $name =~ s/ ([a-z])/ \U$1\E/g; if (length( $name) == 0) { die "Invalid name"; # cannot happen } return $name; }
Compliant Solution
This compliant solution makes the dead code reachable.
sub fix_name { my $name = shift; $name =~ s/^([a-z])/\U$1\E/g; $name =~ s/ ([a-z])/ \U$1\E/g; if (length( $name) == 0) { die "Invalid name"; # cannot happen } return $name; }
Risk Assessment
The presence dead code may indicate logic errors that can lead to unintended program behavior. As a result, resolving dead code can be an in-depth process requiring significant analysis.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC00-PL | low | unlikely | high | P1 | L3 |
Automated Detection
Tool | Diagnostic |
---|---|
Perl::Critic | Subroutines::ProhibitUnusedPrivateSubroutines |
Perl::Critic | ControlStructures::ProhibitUnreachableCode |
Related Guidelines
SEI CERT C Coding Standard | MSC07-C. Detect and remove dead code |
---|---|
SEI CERT C++ Coding Standard | VOID MSC07-CPP. Detect and remove dead code |
Bibliography
[CPAN] | Elliot Shank, Perl-Critic-1.116 Subroutines::ProhibitUnusedPrivateSubroutines, Variables::ProhibitUnreachableCode |
---|
2 Comments
Anonymous
Variables::ProhibitUnreachableCode should be ControlStructures::ProhibitUnreachableCode
David Svoboda
fixed, thanks!