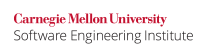
The C Standard, 7.21.9.3 [ISO/IEC 9899:2011], defines the following behavior for fsetpos()
:
The
fsetpos
function sets thembstate_t
object (if any) and file position indicator for the stream pointed to bystream
according to the value of the object pointed to bypos
, which shall be a value obtained from an earlier successful call to thefgetpos
function on a stream associated with the same file.
Invoking the fsetpos()
function with any other values for pos
is undefined behavior.
Noncompliant Code Example
This noncompliant code example attempts to read three values from a file and then set the file position pointer back to the beginning of the file:
#include <stdio.h> #include <string.h> int opener(FILE *file) { int rc; fpos_t offset; memset(&offset, 0, sizeof(offset)); if (file == NULL) { return -1; } /* Read in data from file */ rc = fsetpos(file, &offset); if (rc != 0 ) { return rc; } return 0; }
Only the return value of an fgetpos()
call is a valid argument to fsetpos()
; passing a value of type fpos_t
that was created in any other way is undefined behavior.
Compliant Solution
In this compliant solution, the initial file position indicator is stored by first calling fgetpos()
, which is used to restore the state to the beginning of the file in the later call to fsetpos()
:
#include <stdio.h> #include <string.h> int opener(FILE *file) { int rc; fpos_t offset; if (file == NULL) { return -1; } rc = fgetpos(file, &offset); if (rc != 0 ) { return rc; } /* Read in data from file */ rc = fsetpos(file, &offset); if (rc != 0 ) { return rc; } return 0; }
Risk Assessment
Misuse of the fsetpos()
function can position a file position indicator to an unintended location in the file.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
FIO44-C | Medium | Unlikely | Medium | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 8.1p0 | (customization) | Users can add a custom check for violations of this constraint. |
Compass/ROSE | Can detect common violations of this rule. However, it cannot handle cases in which the value returned by | ||
Helix QAC | 2024.1 | DF4841, DF4842, DF4843 | |
Klocwork | 2024.1 | CERT.FSETPOS.VALUE | |
LDRA tool suite | 9.7.1 | 82 D | Fully implemented |
Parasoft C/C++test | 2023.1 | CERT_C-FIO44-a | Only use values for fsetpos() that are returned from fgetpos() |
Polyspace Bug Finder | R2023b | CERT C: Rule FIO44-C | Checks for invalid file position (rule partially covered) |
PVS-Studio | 7.30 | V1035 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Key here (explains table format and definitions)
Taxonomy | Taxonomy item | Relationship |
---|---|---|
ISO/IEC TS 17961:2013 | Using a value for fsetpos other than a value returned from fgetpos [xfilepos] | Prior to 2018-01-12: CERT: Unspecified Relationship |
Bibliography
[ISO/IEC 9899:2011] | 7.21.9.3, "The fsetpos Function" |