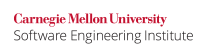
Subclause 7.12.1 of the C Standard [ISO/IEC 9899:2011] defines three types of errors that relate specifically to math functions in math.h
:
A domain error occurs if an input argument is outside the domain over which the mathematical function is defined.
A pole error (also known as a singularity or infinitary) occurs if the mathematical function has an exact infinite result as the finite input argument(s) are approached in the limit
A range error occurs if the mathematical result of the function cannot be represented in an object of the specified type, due to extreme magnitude.
An example of a domain error is the square root of a negative number, such as sqrt(-1.0)
, which has no meaning in real arithmetic. On the other hand, ten raised to the one-millionth power, pow(10., 1e6)
, likely cannot be represented in an implementation's floating-point representation and consequently constitutes a range error. In both cases, the function will return some value, but the value returned is not the correct result of the computation. An example of a pole error is log(0.0)
, which results in negative infinity.
Domain and pole errors can be prevented by carefully bounds-checking the arguments before calling functions and taking alternative action if the bounds are violated.
Range errors usually cannot be prevented because they are dependent on the implementation of floating-point numbers as well as on the function being applied. Instead of preventing range errors, programmers should attempt to detect them and take alternative action if a range error occurs.
The following table lists standard mathematical functions, along with checks that should be performed to ensure a proper input domain, and indicates whether they can also result in range or pole errors, as reported by the C Standard. If a function has a specific domain over which it is defined, the programmer should check its input values, and if a function throws range errors, the programmer should detect whether a range error occurs. The standard math functions not listed in this table, such as atan()
, have no domain restrictions and cannot result in range or pole errors.
Function | Domain | Range | Pole |
---|---|---|---|
|
| No | No |
|
| No | No |
|
| No | No |
|
| No | Yes |
None | Yes | No | |
| None | Yes | No |
| None | Yes | No |
|
| No | Yes |
|
| No | Yes |
|
| Yes | No |
logb(x) | x != 0 | Yes | Yes |
| None | Yes | No |
| None | Yes | No |
| Yes | Yes | |
| No | No | |
| None | Yes | No |
|
| Yes | Yes |
| None | Yes | No |
|
| No | No |
| None | Yes | No |
| None | Yes | No |
| None | Yes | No |
Domain and Pole Checking
The most reliable way to handle domain and pole errors is to prevent them by checking arguments beforehand, as in the following template:
if (/* Arguments that will cause a domain or pole error */) { /* Handle domain or pole error */ } else { /* Perform computation */ }
Range Checking
Range errors cannot usually be prevented, so the most reliable way to handle range errors is to detect when they have occurred and act accordingly.
The exact treatment of error conditions from math functions is quite complicated. Subclause 7.12.1 paragraph 5 of the C Standard [ISO/IEC 9899:2011] defines the following behavior for floating-point overflow:
A floating result overflows if the magnitude of the mathematical result is finite but so large that the mathematical result cannot be represented without extraordinary roundoff error in an object of the specified type. If a floating result overflows and default rounding is in effect, then the function returns the value of the macro
HUGE_VAL
,HUGE_VALF
, orHUGE_VALL
according to the return type, with the same sign as the correct value of the function; if the integer expressionmath_errhandling & MATH_ERRNO
is nonzero, the integer expressionerrno
acquires the valueERANGE
; if the integer expressionmath_errhandling & MATH_ERREXCEPT
is nonzero, the ‘‘overflow’’ floating-point exception is raised.
It is best not to check for errors by comparing the returned value against HUGE_VAL
or 0
for several reasons:
- These are, in general, valid (albeit unlikely) data values.
- Making such tests requires detailed knowledge of the various error returns for each math function.
- There are three different possibilities,
-HUGE_VAL
,0
, andHUGE_VAL
, and you must know which are possible in each case. - Different versions of the library have differed in their error-return behavior.
It is also difficult to check for math errors using errno
because an implementation might not set it. For real functions, the programmer can tell whether the implementation sets errno
by checking whether math_errhandling & MATH_ERRNO
is nonzero. For complex functions, the C Standard, subclause 7.3.2 paragraph 1, simply states that "an implementation may set errno
but is not required to" [ISO/IEC 9899:2011].
The System V Interface Definition (SVID3) [UNIX 1992] provides more control over the treatment of errors in the math library. The user can provide a function named matherr
that is invoked if errors occur in a math function. This function can print diagnostics, terminate the execution, or specify the desired return value. The matherr()
function has not been adopted by C, so its use is not generally portable.
The following error-handing template uses C Standard functions for floating-point errors when the C macro math_errhandling
is defined and indicates that they should be used; otherwise, it examines errno
:
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; /* Call the function */ #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* Handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* Handle range error */ } #endif
See FLP03-C. Detect and handle floating-point errors for more details on how to detect floating-point errors.
Noncompliant Code Example (sqrt()
)
The following noncompliant code determines the square root of x
:
#include <math.h> void func(double x) { double result; result = sqrt(x); }
However, this code may produce a domain error if x
is negative.
Compliant Solution (sqrt()
)
Because this function has domain errors but no range errors, bounds checking can be used to prevent domain errors:
#include <math.h> void func(double x) { double result; if (isless(x, 0.0)) { /* Handle domain error */ } result = sqrt(x); }
Noncompliant Code Example (cosh()
, sinh()
, Range Errors)
This noncompliant code example determines the hyperbolic cosine of x
:
#include <math.h> void func(double x) { double result; result = sinh(x); }
This code may produce a range error if x
has a very large magnitude.
Compliant Solution (cosh()
, sinh()
, Range Errors)
Because this function has no domain errors but may have range errors, the programmer must detect a range error and act accordingly:
#include <errno.h> #include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif void func(double x) { double result; result = sinh(x); #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* Handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* Handle range error */ } #endif }
Noncompliant Code Example (pow()
)
The following noncompliant code raises x
to the power of y
:
#include <math.h> void func(double x, double y) { double result; result = pow(x, y); }
However, this code may produce a domain error if x
is negative and y
is not an integer or if x
is 0 and y
is 0. A domain error or pole error may occur if x
is 0 and y
is negative, and a range error may occur if the result cannot be represented as a double
.
Compliant Solution (pow()
)
Because the pow()
function can produce domain errors, pole errors and range errors, the programmer must first check that x
and y
lie within the proper domain and do not generate a pole error, then detect whether a range error occurs and act accordingly:
#include <errno.h> #include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif void func(double x, double y) { #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; double result; if (((x == 0.0f) && islessequal(y, 0.0)) || isless(x, 0.0)) { /* Handle domain or pole error */ } result = pow(x, y); #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* Handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* Handle range error */ } #endif }
Risk Assessment
Failure to prevent or detect domain and range errors in math functions may result in unexpected results.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
FLP32-C | Medium | Probable | Medium | P8 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
5.0 |
| Can detect violations of this rule with CERT C Rule Pack |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Bibliography
[ISO/IEC 9899:2011] | Subclause 7.3.2, "Conventions" Subclause 7.12.1, "Treatment of Error Conditions" |
[Plum 1985] | Rule 2-2 |
[Plum 1989] | Topic 2.10, "conv—Conversions and Overflow" |
[UNIX 1992] | System V Interface Definition (SVID3) |