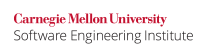
An unsafe function-like macro is one that, when expanded, evaluates its argument more than once or doesn't evaluate it at all. Contrasted with function calls, which always evaluate each of their arguments exactly once, unsafe function-like macros often have unexpected and surprising effects and lead to subtle, hard to find defects (see PRE31-C. Avoid side-effects in arguments to unsafe macros). Consequently, every function-like macro should evaluate each of its arguments exactly once. Alternatively and preferably, defining function-like macros should be avoided in favor of inline functions (see PRE00-C. Prefer inline or static functions to function-like macros).
Noncompliant Code Example (multiple argument evaluation)
The most severe problem with unsafe function-like macros is side effects of macro arguments, as shown in this noncompliant code example.
#define ABS(x) (((x) < 0) ? -(x) : (x)) void f(int n) { int m; m = ABS(++n); /* undefined behavior */ /* ... */ }
The invocation of the ABS()
macro in this noncompliant code example expands to the code below. Since the resulting expression modifies an object more than once, its behavior is undefined (see EXP30-C. Do not depend on order of evaluation between sequence points).
m = (((++n) < 0) ? -(++n) : (++n)); /* undefined behavior */
Compliant Solution (inline function)
A possible and preferable compliant solution is to define an inline function with equivalent but safe semantics:
inline int Abs(x) { return x < 0 ? -x : x; }
Compliant Solution (language extension)
Some implementations provide language extensions that make it possible to define safe function-like macros such as the macro ABS()
above that would otherwise require evaluating their arguments more than once. For example, the gcc extension Statements and Declarations in Expressions makes it possible to implement the macro ABS()
in a safe way. Note, however, that since relying on implementation-defined extensions introduces undesirable platform dependencies that may make the resulting code non-portable, such solutions should be avoided in favor of portable ones wherever possible (see MSC14-C. Do not introduce unnecessary platform dependencies).
#define ABS(x) ({int __x = (x); __x < 0 ? -__x : __x; })
Risk Assessment
Defining an unsafe macro leads invocations of the macro with an argument that has side effects, causing those side effects to occur more than once. This can lead to unexpected or undefined program behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
PRE33-C |
low |
probable |
low |
P6 |
L2 |
Automated Detection
Unknown.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as PRE33-CPP. Never invoke an unsafe macro with arguments containing assignment, increment, decrement, volatile access, or function call.
References
[[ISO/IEC 9899:1999]] Section 5.1.2.3, "Program execution"
01. Preprocessor (PRE) PRE32-C. Do not use preprocessor directives inside macro arguments