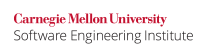
The C99 standard [[ISO/IEC 9899:1999]] introduces flexible array members into the language. While flexible array members are a useful addition they should be properly understood and used with care.
Flexible array members are defined in Section 6.7.2.1, paragraph 16 of the C99 standard as follows,
As a special case, the last element of a structure with more than one named member may have an incomplete array type; this is called a flexible array member. In most situations, the flexible array member is ignored. In particular, the size of the structure is as if the flexible array member were omitted except that it may have more trailing padding than the omission would imply. However, when a . (or ->) operator has a left operand that is (a pointer to) a structure with a flexible array member and the right operand names that member, it behaves as if that member were replaced with the longest array (with the same element type) that would not make the structure larger than the object being accessed; the offset of the array shall remain that of the flexible array member, even if this would differ from that of the replacement array. If this array would have no elements, it behaves as if it had one element but the behavior is undefined if any attempt is made to access that element or to generate a pointer one past it.
The following is an example of a structure that contains a flexible array member,
struct flexArrayStruct { int num; int data[]; };
This definition means that, when allocating storage space, only the first member, num
, is considered. Consequently, the result of accessing the member data
of a variable of type struct flexArrayStruct
is undefined. To avoid the potential for undefined behavior, structures that contain a flexible array member should always be accessed with a pointer as shown in the following code example.
struct flexArrayStruct *structP; size_t array_size; size_t i; /* Initialize array_size */ /* Space is allocated for the struct */ structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (structP == NULL) { /* Handle malloc failure */ } structP->num = 0; /* * Access data[] as if it had been allocated * as data[array_size] */ for (i = 0; i < array_size; i++) { structP->data[i] = 1; }
Structures with flexible array members can be used to produce code with defined behavior. However, some restrictions apply:
- The incomplete array type must be the last element within the structure.
- There cannot be an array of structures that contain flexible array members.
- Structures that contain a flexible array member cannot be used as a member in the middle of another structure.
Noncompliant Code Example (Use Flexible Array Members)
When using C99 compliant compilers the one element array hack described above should not be used. In this noncompliant code, just such an array is used where a flexible array member should be used instead.
struct flexArrayStruct { int num; int data[1]; }; /* ... */ struct flexArrayStruct *flexStruct; size_t array_size; size_t i; /* Initialize array_size */ /* Dynamically allocate memory for the structure */ flexStruct = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * (array_size - 1)); if (flexStruct == NULL) { /* Handle malloc failure */ } /* Initialize structure */ flexStruct->num = 0; for (i = 0; i < array_size; i++) { flexStruct->data[i] = 0; }
As described above, the problem with this code is that strictly speaking the only member that is guaranteed to be valid is flexStruct->data[0]
. Unfortunately, when using compilers that do not support the C99 standard in full, or at all, this approach may be the only solution. Microsoft Visual Studio 2005, for example, does not implement the C99 syntax.
Compliant Solution (Use Flexible Array Members)
Fortunately, when working with C99 compliant compilers, the solution is simple - remove the 1 from the array declaration and adjust the size passed to the malloc() call accordingly. In other words, use flexible array members.
struct flexArrayStruct { int num; int data[]; }; /* ... */ struct flexArrayStruct *flexStruct; size_t array_size; size_t i; /* Initialize array_size */ /* Dynamically allocate memory for the structure */ flexStruct = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (flexStruct == NULL) { /* Handle malloc failure */ } /* Initialize structure */ flexStruct->num = 0; for (i = 0; i < array_size; i++) { flexStruct->data[i] = 0; }
In this case, the structure will be treated as if the member data[]
had been declared to be data[array_size].
Noncompliant Code Example (Declaration)
When using structures with a flexible array member you should never directly declare an instance of the structure. In this noncompliant code, a variable of type struct flexArrayStruct is declared.
struct flexArrayStruct flexStruct; size_t array_size; size_t i; /* Initialize array_size */ /* Initialize structure */ flexStruct.num = 0; for (i = 0; i < array_size; i++) { flexStruct.data[i] = 0; }
The problem with this code is that the flexArrayStruct
does not actually reserve space for the integer array data - it can't as the size hasn't been specified. Consequently, while initializing the num member to zero is allowed, attempting to write even one value into data (that is, data[0]
) will likely overwrite memory not owned by the structure.
Compliant Code Example (Declaration)
The solution is to always declare pointers to structures containing a flexible array member and dynamically allocate memory for them. The following code snippet illustrates this.
struct flexArrayStruct *flexStruct; size_t array_size; size_t i; /* Initialize array_size */ /* Dynamically allocate memory for the structure */ flexStruct = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (flexStruct == NULL) { /* Handle malloc failure */ } /* Initialize structure */ flexStruct->num = 0; for (i = 0; i < array_size; i++) { flexStruct->data[i] = 0; }
In this code snippet the resolves the issue by declaring a pointer to flexArrayStruct
and then dynamically allocating memory for the pointer to point to. In this case it is acceptable to access the elements of the data[]
member as described in C99 Section 6.7.2.1, paragraph 16.
Noncompliant Code Example (Copying)
When using structures with a flexible array member you should never directly copy an instance of the structure. This noncompliant code attempts to replicate a copy of struct flexArrayStruct
.
struct flexArrayStruct *flexStructA; struct flexArrayStruct *flexStructB; size_t array_size; size_t i; /* Initialize array_size */ /* Allocate memory for flexStructA */ /* Allocate memory for flexStructB */ /* Initialize flexStructA */ /* ... */ *flexStructB = *flexStructA;
The problem with this code is that when the structure is copied the size of the flexible array member is not considered and only the first member of the structure, num
, is copied.
Compliant Solution (Copying)
This compliant solution uses memcpy()
to properly copy the content of flexStructA
into flexStructB
.
struct flexArrayStruct *flexStructA; struct flexArrayStruct *flexStructB; size_t array_size; size_t i; /* Initialize array_size */ /* Allocate memory for flexStructA */ /* Allocate memory for flexStructB */ /* Initialize flexStructA */ /* ... */ memcpy(flexStructB, flexStructA, (sizeof(struct flexArrayStruct) + sizeof(int) * array_size));
In this case the copy is explicit and the flexible array member is accounted for and copied as well.
Noncompliant Code Example (Reference)
When using structures with a flexible array member you should never directly pass an instance of the structure in a function call. In this noncompliant code, the flexible array structure is passed directly to a function which tries to print the array elements.
void print_array(struct flexArrayStruct structP) { size_t i; printf("Array is: "); for (i = 0; i < structP.num; i++) { printf("%d", structP.data[i]); } printf("\n"); } struct flexArrayStruct *structP; size_t array_size; size_t i; /* initialize array_size */ /* space is allocated for the struct */ structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (structP == NULL) { /* Handle malloc failure */ } structP->num = array_size; for (i = 0; i < array_size; i++) { structP->data[i] = i; } print_array(*structP);
The problem with this code is that passing the structure directly to the function actually makes a copy of the structure. This copied fails for the same reason as the copy example above.
Compliant Solution (Reference)
Never allow a structure with a flexible array member to be passed directly in a function call. The above code can be fixed by changing the function to accept a pointer to the structure.
void print_array(struct flexArrayStruct *structP) { size_t i; printf("Array is: "); for (i = 0; i < structP->num; i++) { printf("%d", structP->data[i]); } printf("\n"); } struct flexArrayStruct *structP; size_t array_size; size_t i; /* initialize array_size */ /* space is allocated for the struct */ structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (structP == NULL) { /* Handle malloc failure */ } structP->num = array_size; for (i = 0; i < array_size; i++) { structP->data[i] = i; } print_array(structP);
Risk Assessment
Failure to use structures with flexible array members correctly can result in undefined behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MEM33-C |
low |
unlikely |
low |
P3 |
L3 |
Automated Detection
Compass/ROSE can detect some violations of this rule. In particular, it warns if the last element of a struct is an array with a small index (0 or 1).
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]]
[JTC1/SC22/WG14 N791]
MEM32-C. Detect and handle memory allocation errors 08. Memory Management (MEM)