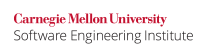
Bitwise shifts include left shift operations of the form shift-expression <<
additive-expression and right shift operations of the form shift-expression >>
additive-expression. The integer promotions are performed on the operands, each of which has integer type. The type of the result is that of the promoted left operand. If the value of the right operand is negative or is greater than or equal to the width of the promoted left operand, the behavior is undefined.
Non-Compliant Code Example (left shift, signed type)
The result of E1 << E2
is E1
left-shifted E2
bit positions; vacated bits are filled with zeros. If E1
has a signed type and nonnegative value and E1 * 2 E2
is representable in the result type, then that is the resulting value; otherwise, the behavior is undefined.
The following code can result in undefined behavior because there is no check to ensure that left and right operands have nonnegative values and that the right operand is greater than or equal to the width of the promoted left operand.
int si1, si2, sresult; sresult = si1 << si2;
Compliant Solution (left shift, signed type)
This compliant solution eliminates the possibility of undefined behavior resulting from a left shift operation on signed and unsigned integers. Smaller sized integers are promoted according to the integer promotion rules [[INT02-A]].
int si1, si2, sresult; if ( (si1 < 0) || (si2 < 0) || (si2 >= sizeof(int)*CHAR_BIT) || si1 > (INT_MAX >> si2) ) { /* handle error condition */ } else { sresult = si1 << si2; }
In C99, the CHAR_BIT
macro defines the number of bits for the smallest object that is not a bit-field (byte). A byte, therefore, contains CHAR_BIT
bits.
Non-Compliant Code Example (left shift, unsigned type)
The result of E1 << E2
is E1
left-shifted E2
bit positions; vacated bits are filled with zeros. According to C99, if E1
has an unsigned type, the value of the result is E1 * 2 E2
, reduced modulo one more than the maximum value representable in the result type. Although C99 specifies modulo behavior for unsigned integers, unsigned integer overflow frequently results in unexpected values and resultant security vulnerabilities (see [[INT32-C]]). Consequently, unsigned overflow is generally non-compliant and E1 * 2 E2
must be representable in the result type. Modulo behavior is allowed if the conditions in the exception section are met.
The following code can result in undefined behavior because there is no check to ensure that the right operand is greater than or equal to the width of the promoted left operand.
unsigned int ui1, ui2, uresult; uresult = ui1 << ui2;
Compliant Solution (left shift, unsigned type)
This compliant solution eliminates the possibility of undefined behavior resulting from a left shift operation on unsigned integers. Example solutions are provided for the fully compliant case (unsigned overflow is prohibited) and the exceptional case (modulo behavior is allowed).
unsigned int ui1, ui2, uresult; unsigned int mod1, mod2; /* modulo behavior is allowed on mod1 and mod2 by exception */ if ( (ui2 >= sizeof(unsigned int)*CHAR_BIT) || (ui1 > (UINT_MAX >> ui2))) ) { /* handle error condition */ } else { uresult = ui1 << ui2; } if (mod2 >= sizeof(unsigned int)*CHAR_BIT) { /* handle error condition */ } else { uresult = mod1 << mod2; /* modulo behavior is allowed by exception */ }
Non-Compliant Code Example (right shift)
The result of E1 >> E2
is E1
right-shifted E2
bit positions. If E1
has an unsigned type or if E1
has a signed type and a nonnegative value, the value of the result is the integral part of the quotient of E1 / 2 E2
. If E1
has a signed type and a negative value, the resulting value is implementation-defined and may be either an arithmetic (signed) shift:
or a logical (unsigned) shift:
This non-compliant code example fails to test whether the right operand is negative or is greater than or equal to the width of the promoted left operand, allowing undefined behavior.
int si1, si2, sresult; unsigned int ui1, ui2, uresult; sresult = si1 >> si2; uresult = ui1 >> ui2;
Making assumptions about whether a right shift is implemented as an arithmetic (signed) shift or a logical (unsigned) shift can also lead to vulnerabilities (see [[INT13-A]]).
Compliant Solution (right shift)
This compliant solution tests the suspect shift operation to guarantee there is no possibility of unsigned overflow.
int si1, si2, sresult; unsigned int ui1, ui2, result; if ( (si2 < 0) || (si2 >= sizeof(int)*CHAR_BIT) ) { /* handle error condition */ } else { sresult = si1 >> si2; } if (ui2 >= sizeof(unsigned int)*CHAR_BIT) { /* handle error condition */ } else { uresult = ui1 >> ui2; }
Exceptions
Unsigned integers can be allowed to exhibit modulo behavior if and only if
- the variable declaration is clearly commented as supporting modulo behavior
- each operation on that integer is also clearly commented as supporting modulo behavior
If the integer exhibiting modulo behavior contributes to the value of an integer not marked as exhibiting modulo behavior, the resulting integer must obey this rule.
Risk Assessment
Improper range checking can lead to buffer overflows and the execution of arbitary code by an attacker.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT36-C |
2 (medium) |
2 (probable) |
2 (medium) |
P8 |
L2 |
Examples of vulnerabilities resulting from the violation of this rule can be found on the CERT web site.
References
A test program for this rule is available.
[[Dowd 06]] Chapter 6, "C Language Issues"
[[ISO/IEC 9899-1999]] Section 6.5.7, "Bitwise shift operators"
[[Seacord 05]] Chapter 5, "Integers"
[[Viega 05]] Section 5.2.7, "Integer overflow"
[[ISO/IEC 03]] Section 6.5.7, "Bitwise shift operators"