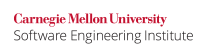
Do not hard-code the size of a type into an application. Because of alignment, padding, and differences in basic types (e.g., 32-bit versus 64-bit pointers), the size of most types can vary between compilers and even version of the same compiler. Using the sizeof
operator to determine sizes improves the clarity of what is meant and ensures that changes between compilers or version will not affect the code.
Type alignment requirements can also affect the size of structs. Consider the following structure:
struct s { int i; double d; };
Depending on the compiler and platform, this structure could be any of a variety of sizes. Assuming 32-bit integers and 64-bit doubles, the size might be 12 or 16 bytes, depending on alignment rules.
Non-Compliant Coding Example
This non-compliant example demonstrates the incorrect way to declare a triangular array of integers. On a platform with 64-bit integers, the loop will access memory outside the allocated memory section.
/* assuming 32-bit pointer, 32-bit integer */ size_t i; int ** triarray = calloc(100, 4); if (triarray == NULL) { /* handle error */ } for (i = 0; i < 100; i++) { triarray[i] = calloc(i, 4); if (triarray[i] == NULL) { /* handle error */ } }
Compliant Solution
The above example can be fixed by replacing the hard-coded value 4
with the size of the type using sizeof
.
size_t i; int **triarray = calloc(100, sizeof(int *)); if (!triarray) { /* handle error */ } for (i = 0; i < 100; i++) { triarray[i] = calloc(i, sizeof(int)); if (!triarray[i]) { /* handle error */ } }
Risk Assessment
If non-compliant code is ported to a different platform, it could introduce a buffer or stack overflow vulnerability.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXPxx-A |
3 (high) |
1 (unlikely) |
2 (medium) |
P6 |
L2 |
References
[[ISO/IEC 9899-1999]] Section 6.2.6, "Representations of types," and Section 6.5.3.4, "The sizeof operator"