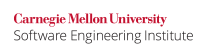
When writing a library, each exposed functions should perform a validity check on its parameters. Validity check allows the library to survive improper usage, enabling an application using the library to likewise survive and often simplifies the task of determining the condition that caused the illegal parameter.
Non-Compliant Coding Example
For these examples, a library exposes an API as follows.
/* sets some internal state in the library */ extern int setfile(FILE *file); /* performs some action using the file passed earlier */ extern int usefile();
In this non-compliant example, setfile
and usefile
do not validate their parameters. It is possible that an invalid file pointer may be used by the library, possibly corrupting the library's internal state and exposing a vulnerability.
static FILE *myFile; int setfile(FILE *file) { myFile = file; return 0; } int usefile() { /* perform some action here */ return 0; }
The vulnerability is more severe if the internal state references sensitive or system-critical data.
Compliant Solution
Validating the function parameters and verifying the internal state leads to consistency of program execution and may eliminate potential vulnerabilities, presuming the application using the library properly uses the library.
static FILE *myFile; int setfile(FILE *file) { if (file && !ferror(file) && !feof(file)) { myFile = file; return 0; } myFile = NULL; return -1; } int usefile() { if (!myFile) return -1; /* perform other checks if needed, return error condition */ /* perform some action here */ return 0; }
Risk Assessment
The most likely result of ignoring this recommendation is an access violation or a data integrity violation. Such a scenario is indicative of a flaw in the manner in which the library is used by the calling code. However, it may still be the library itself that is the vector by which the calling code's vulnerability is exploited.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSCxx-A |
1 (low) |
1 (unlikely) |
1 (low) |
P1 |
L3 |
References
Apple, Inc. Secure Coding Guide: Application Interfaces That Enhance Security. Retrieved Apr 26, 2007.