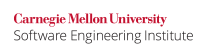
Prevent or detect domain errors and range errors in math functions before using the results in further computations. Most of the math functions can fail if they are given arguments that do not produce a proper result. For example, the square root of a negative number, such as sqrt(-1.0)
, has no meaning in real arithmetic. This is known as a domain error; the argument is outside the domain over which the function is defined. For another example, ten raised to the one-millionth power, pow(10., 1e6)
, cannot be represented in any (current) floating point representation. This is known as a range error; the result cannot be represented as a proper double
number. In all such error cases, the function will return some value, but the value returned is not the correct result of the computation.
Math errors can be prevented by carefully bounds-checking the arguments before calling functions. In particular, the following functions should be bounds checked as follows:
Function |
Bounds-checking |
---|---|
-1 <= x && x <= 1 |
|
x != 0 || y != 0 |
|
x >= 0 |
|
x != 0 || y > 0 |
|
x >= 0 |
The calling function should take alternative action if these bounds are violated.
acos( x ), asin( x )
Non-Compliant Code Example
This code may produce a domain error if the argument is not in the range [-1, +1].
float x, result; result = acos(x);
Compliant Solution
This code uses bounds checking to ensure that there is not a domain error.
float x, result; if ( islessequal(x,-1) || isgreaterequal(x, 1) ){ /* handle domain error */ } result = acos(x);
atan2( y, x )
Non-Compliant Code Example
This code may produce a domain error if both x and y are zero.
float x, y, result; result = atan2(y, x);
Compliant Solution
This code tests the arguments to ensure that there is not a domain error.
float x, y, result; if ( (x == 0.f) && (y == 0.f) ) { /* handle domain error */ } result = atan2(y, x);
log( x ), log10( x )
Non-Compliant Code Example
This code may produce a domain error if x is negative and a range error if x is zero.
float result, x; result = log(x);
Compliant Solution
This code tests the suspect arguments to ensure that no domain or range errors are raised.
float result, x; if (islessequal(x, 0)) { /* handle domain and range errors */ } result = log(x);
pow( x, y )
Non-Compliant Code Example
This code may produce a domain error if x is zero and y less than or equal to zero. A range error may also occur if x is zero and y is negative.
float x, y, result; result = pow(x, y);
Compliant Solution
This code tests x and y to ensure that there will be no range or domain errors.
float x, y, result; if ( (x == 0.f) && islessequal(y, 0) ) { * handle domain error condition */ } result = pow(x, y);
sqrt( x )
Non-Compliant Code Example
This code may produce a domain error if x is negative.
float x, result; result = sqrt(x);
Compliant Solution
This code tests the suspect argument to ensure that no domain error is raised.
float x, result; if (isless(x, 0)){ /* handle domain error */ } result = sqrt(x);
Non-Compliant Coding Example (Error Checking)
The exact treatment of error conditions from math functions is quite complicated (see C99 Section 7.12.1, "Treatment of error conditions").
The sqrt()
function returns zero when given a negative argument. The pow()
function returns a very large number (appropriately positive or negative) on an overflow range error. This very large number is defined in <math.h>
as described by C99 [[ISO/IEC 9899-1999]]:
The macro
HUGE_VALexpands to a positive double constant expression, not necessarily representable as a
float. The macrosHUGE_VALF HUGE_VALLare respectively float and long double analogs of
HUGE_VAL
.
It is best not to check for errors by comparing the returned value against HUGE_VAL
or zero for several reasons. First of all, these are in general valid (albeit unlikely) data values. Secondly, making such tests requires detailed knowledge of the various error returns for each math function. Also, there are three different possibilities, -HUGE_VAL, 0, and HUGE_VAL, and you must know which are possible in each case. Finally, different versions of the library have differed in their error-return behavior.
It is also difficult to check for math errors using errno
because an implementation might not set it. For real functions, the programmer can test whether the implementation sets errno
because in that case math_errhandling & MATH_ERRNO
is nonzero. For complex functions, the C Standard Section 7.3.2 simply states that setting errno
is optional.
Compliant Solution (Error Checking)
The most reliable way to test for errors is by checking arguments beforehand, as in the following compliant solution:
if (/* arguments will cause a domain or range error */) { /* handle the error */ } else { /* perform computation */ }
Implementation Details
System V Interface Definition, Third Edition
The System V Interface Definition, Third Edition (SVID3) provides more control over the treatment of errors in the math library. The user can provide a function named matherr
that is invoked if errors occur in a math function. This function could print diagnostics, terminate the execution, or specify the desired return-value. The matherr()
function has not been adopted by the C standard, so its use is not generally portable.
Risk Assessment
Failure to properly verify arguments supplied to math functions may result in unexpected results.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FLP32-C |
2 (medium) |
2 (probable) |
2 (medium) |
P8 |
L2 |
Automated Detection
Fortify SCA Version 5.0 with CERT C Rule Pack can detect violations of this rule.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 7.3, "Complex arithmetic <complex.h>"
[[ISO/IEC 9899-1999]] Section 7.12, "Mathematics <math.h>"
[[Plum 85]] Rule 2-2
[[Plum 89]] Topic 2.10, "conv - conversions and overflow"
FLP31-C. Do not call functions expecting real values with complex values 05. Floating Point (FLP) FLP33-C. Convert integers to floating point for floating point operations