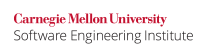
One of the problems with arrays is determining the size. The sizeof
operator yields the size (in bytes) of its operand, which may be an expression or the parenthesized name of a type.
Non-Compliant Code Example
In this example, the sizeof
operator returns the size of the pointer, not the size of the block of space the pointer refers to. As a result the call to malloc()
returns a pointer to a block of memory the size of a pointer. When the strcpy()
is called, a heap buffer overflow will occur.
char *src = "hello, world"; char *dest = malloc(sizeof(src)); strcpy(dest, src);
Compliant Solution
Fixing this issue requires the programmer to recognize and understand how sizeof
works. In this case if, changing the type of src
to a character array will correct the problem.
char src[] = "hello, world"; char *dest = malloc(sizeof(src)); strcpy(dest, src);
Non-Compliant Code Example
The sizeof
operator can be used to compute the number of elements in an array as follows: sizeof (dis) / sizeof (dis[0])
. The sizeof
operator can also be used to calculate the size of variable length arrays. In the case of a variable length array, the operand is evaluated at runtime. Extreme care must be taken when using this particular programming idiom, however.
void f(int a[]) { int i; for (i = 0; i < sizeof (a) / sizeof (a[0]); i++) { a[i] = 21; } } int main(void) { int dis[12]; f(dis); }
In this example, sizeof (a) / sizeof (a[0])
evaluates to sizeof(int*)/sizeof(int)
because int a[]
is equivalent to int *a
in the function declaration. This allows f()
to be passed an array of arbitrary length.
Compliant Solution
This problem can be fixed by passing the size as a separate argument, as shown in this example:
void g(int a[], int size) { int i; for (i = 0; i < size; i++) { a[i] = 21; } } int main(void) { int dis[12]; g(dis, sizeof (dis) / sizeof (dis[0])); }
Care must be taken to ensure that the size is valid for the array. If these parameters can be manipulated by an attacker, this function will almost always result in an exploitable vulnerability.
References
- ISO/IEC 9899-1999 Section 6.7.5.2 Array declarators