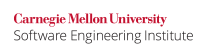
The size_t
type is the unsigned integer type of the result of the sizeof
operator. The underlying representation of variables of type size_t
are guaranteed to be of sufficient precision to represent the size of an object. The limit of size_t
is specified by the SIZE_MAX
macro.
Any variable that is used to represent the size of an object including, but not limited to, integer values used as sizes, indices, loop counters, and lengths should be declared as size_t
.
Non-Compliant Code Example
In this example, the dynamically allocated buffer referenced by p will overflow for values of n > INT_MAX
.
char *copy(size_t n, char *str) { int i; char *p = malloc(n); for ( i = 0; i < n; ++i ) { p[i] = *str++; } return p; } char *p = copy(20, "hi there");
Compliant Solution
Declaring i to be of type size_t
eliminates the possible integer overflow condition.
char *copy(size_t n, char *str) { size_t i; char *p = malloc(n); for ( i = 0; i < n; ++i ) { p[i] = *str++; } return p; } char *p = copy(20, "hi there");
Non-Compliant Code Example
The user defined function calc_size
(not shown) is used to calculate the size of the string other_string
. The result of calc_size
is a signed int
returned into str_size
. Given that there is no check on str_size
, it is impossible to tell whether the result of calc_size
is an appropriate parameter for malloc, that is, a positive integer that can be properly represented by a signed int
type.
int str_size = calc_size(other_string); char *str_copy = malloc(str_size);
Compliant Solution
By changing str_size
to a variable of type size_t
, it can be ensured that the call to malloc()
is, at the least, supplied a non-negative number.
size_t str_size = calc_size(other_string); char *str_copy = malloc(str_size);
Non-Compliant Code Example
Add an example using size_t as an index
References
- ISO/IEC 9899-1999 Section 7.17 Common definitions <stddef.h>
- ISO/IEC 9899-1999 Section 7.20.3 Memory management functions