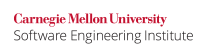
C library functions that make changes to arrays or objects take at least two arguments: a pointer to the array or object and an integer indicating the number of elements or bytes to be manipulated. If improper arguments are supplied to such a function, it might cause the function to form a pointer that does not point into or just past the end of the object, resulting in undefined behavior.
The C Standard identifies the following distinct situations in which undefined behavior (UB) can arise as a result of invalid pointer operations:
UB | Description |
---|---|
The pointer passed to a library function array parameter does not have a value such that all address computations and object accesses are valid. |
For the purposes of this rule, the element count of a pointer is the size of the object to which it points, expressed by the number of elements that are valid to access.
In the following code,
int arr[5]; int *p = arr; unsigned char *p2 = (unsigned char *)arr; unsigned char *p3 = arr + 2; void *p4 = arr;
the element count of the pointer p
is sizeof(arr) / sizeof(arr[0])
, that is, 5
. The element count of the pointer p2
is sizeof(arr)
, that is, 20
, on platforms where sizeof(int) == 4
. The element count of the pointer p3
is 12
on platforms where sizeof(int) == 4
, because p3
points two elements past the start of the array arr
. The element count of p4
is treated as though it were unsigned char *
instead of void *
, so it is the same as p2
.
Library Functions That Take a Pointer and Integer
The following standard library functions take a pointer argument and a size argument, with the constraint that the pointer must point to a valid memory object of at least the number of elements indicated by the size argument.
fgets() | fgetws() | mbstowcs() 1 | wcstombs() 1 |
mbrtoc16() 2 | mbrtoc32() 2 | mbsrtowcs() 1 | wcsrtombs() 1 |
mbtowc() 2 | mbrtowc() 1 | mblen() | mbrlen() |
memchr() | wmemchr() | memset() | wmemset() |
strftime() | wcsftime() | strxfrm()1 | wcsxfrm()1 |
strncat()2 | wcsncat()2 | snprintf() | vsnprintf() |
swprintf() | vswprintf() | setvbuf() | tmpnam_s() |
snprintf_s() | sprintf_s() | vsnprintf_s() | vsprintf_s() |
gets_s() | getenv_s() | wctomb_s() | mbstowcs_s()3 |
wcstombs_s()3 | memcpy_s()3 | memmove_s()3 | strncpy_s()3 |
strncat_s()3 | strtok_s()2 | strerror_s() | strnlen_s() |
asctime_s() | ctime_s() | snwprintf_s() | swprintf_s() |
vsnwprintf_s() | vswprintf_s() | wcsncpy_s()3 | wmemcpy_s()3 |
wmemmove_s()3 | wcsncat_s()3 | wcstok_s()2 | wcsnlen_s() |
wcrtomb_s() | mbsrtowcs_s()3 | wcsrtombs_s()3 | memset_s()4 |
1 Takes two pointers and an integer, but the integer specifies the element count only of the output buffer, not of the input buffer.
2 Takes two pointers and an integer, but the integer specifies the element count only of the input buffer, not of the output buffer.
3 Takes two pointers and two integers; each integer corresponds to the element count of one of the pointers.
4 Takes a pointer and two size-related integers; the first size-related integer parameter specifies the number of bytes available in the buffer; the second size-related integer parameter specifies the number of bytes to write within the buffer.
For calls that take a pointer and an integer size, the given size should not be greater than the element count of the pointer.
Noncompliant Code Example (Element Count)
In this noncompliant code example, the incorrect element count is used in a call to wmemcpy()
. The sizeof
operator returns the size expressed in bytes, but wmemcpy()
uses an element count based on wchar_t *
.
#include <string.h> #include <wchar.h> static const char str[] = "Hello world"; static const wchar_t w_str[] = L"Hello world"; void func(void) { char buffer[32]; wchar_t w_buffer[32]; memcpy(buffer, str, sizeof(str)); wmemcpy(w_buffer, w_str, sizeof(w_str)); }
Compliant Solution (Element Count)
When using functions that operate on pointed-to regions, programmers must always express the integer size in terms of the element count expected by the function. For instance, memcpy()
expects the element count expressed in terms of void *
, but wmemcpy()
expects the element count expressed in terms of wchar_t *
. Instead of using the sizeof
operator, calls to return the number of elements in the string are used, which matches the expected element count for the copy functions.
#include <string.h> #include <wchar.h> static const char str[] = "Hello world"; static const wchar_t w_str[] = L"Hello world"; void func(void) { char buffer[32]; wchar_t w_buffer[32]; memcpy(buffer, str, strlen(str) + 1); wmemcpy(w_buffer, w_str, wcslen(w_str) + 1); }
Noncompliant Code Example (Pointer + Integer)
This noncompliant code example assigns a value greater than the number of bytes of available memory to n
, which is then passed to memset()
:
#include <stdlib.h> #include <string.h> void f1(size_t nchars) { char *p = (char *)malloc(nchars); /* ... */ const size_t n = nchars + 1; /* ... */ memset(p, 0, n); }
Compliant Solution (Pointer + Integer)
This compliant solution ensures that the value of n
is not greater than the number of bytes of the dynamic memory pointed to by the pointer p
:
#include <stdlib.h> #include <string.h> void f1(size_t nchars) { char *p = (char *)malloc(nchars); /* ... */ const size_t n = nchars; /* ... */ memset(p, 0, n); }
Noncompliant Code Example (Pointer + Integer)
In this noncompliant code example, the element count of the array a
is ARR_SIZE
elements. Because memset()
expects a byte count, the size of the array is scaled incorrectly by sizeof(int)
instead of sizeof(float)
, which can form an invalid pointer on architectures where sizeof(int) != sizeof(float)
.
#include <string.h> void f2() { const size_t ARR_SIZE = 4; float a[ARR_SIZE]; const size_t n = sizeof(int) * ARR_SIZE; void *p = a; memset(p, 0, n); }
Compliant Solution (Pointer + Integer)
In this compliant solution, the element count required by memset()
is properly calculated without resorting to scaling:
#include <string.h> void f2() { const size_t ARR_SIZE = 4; float a[ARR_SIZE]; const size_t n = sizeof(a); void *p = a; memset(p, 0, n); }
Library Functions That Take Two Pointers and an Integer
The following standard library functions take two pointer arguments and a size argument, with the constraint that both pointers must point to valid memory objects of at least the number of elements indicated by the size argument.
| wmemcpy() | memmove() | wmemmove() |
strncpy() | wcsncpy() | memcmp() | wmemcmp() |
strncmp() | wcsncmp() | strcpy_s() | wcscpy_s() |
strcat_s() | wcscat_s() |
For calls that take two pointers and an integer size, the given size should not be greater than the element count of either pointer.
Noncompliant Code Example (Two Pointers + One Integer)
In this noncompliant example, a diagnostic is required because the value of n
is not computed correctly, allowing a possible write past the end of the object referenced by p
:
#include <string.h> void f4(char p[], const char *q) { const size_t n = sizeof(p); if ((memcpy(p, q, n)) == p) { /* ... */ } }
This example also violates ARR01-C. Do not apply the sizeof operator to a pointer when taking the size of an array.
Compliant Solution (Two Pointers + One Integer)
This compliant solution ensures that n
is equal to the size of the character array:
#include <string.h> void f4(char p[], const char *q, size_t size_p) { const size_t n = size_p; if ((memcpy(p, q, n)) == p) { /* ... */ } }
Library Functions That Take a Pointer and Two Integers
The following standard library functions take a pointer argument and two size arguments, with the constraint that the pointer must point to a valid memory object containing at least as many bytes as the product of the two size arguments.
bsearch() | bsearch_s() | qsort() | qsort_s() |
fread() | fwrite() | |
For calls that take a pointer and two integers, one integer represents the number of bytes required for an individual object, and a second integer represents the number of elements in the array. The resulting product of the two integers should not be greater than the element count of the pointer were it expressed as an unsigned char *
.
Noncompliant Code Example (One Pointer + Two Integers)
This noncompliant code example allocates a variable number of objects of type struct obj
. The function checks that num_objs
is small enough to prevent wrapping, in compliance with INT30-C. Ensure that unsigned integer operations do not wrap. The size of struct obj
is assumed to be 8 bytes to account for padding. However, the padding is dependent on the target architecture as well as compiler settings, so this object size may be incorrect resulting in an incorrect element count.
#include <stdint.h> #include <stdio.h> struct obj { char c; int i; }; void func(FILE *f, struct obj *objs, size_t num_objs) { const size_t obj_size = 8; if (num_objs > (SIZE_MAX / obj_size) || num_objs != fwrite(objs, obj_size, num_objs, f)) { /* Handle error */ } }
Compliant Solution (One Pointer + Two Integers)
This compliant solution uses the sizeof
operator to correctly provide the object size and num_objs
to provide the element count:
#include <stdint.h> #include <stdio.h> struct obj { char c; int i; }; void func(FILE *f, struct obj *objs, size_t num_objs) { if (num_objs > (SIZE_MAX / sizeof(*objs)) || num_objs != fwrite(objs, sizeof(*objs), num_objs, f)) { /* Handle error */ } }
Noncompliant Code Example (One Pointer + Two Integers)
In this noncompliant code example, the function f()
calls fread()
to read nitems
of type wchar_t
, each size
bytes in size, into an array of BUFFER_SIZE
elements, wbuf
. However, the expression used to compute the value of nitems
fails to account for the fact that, unlike the size of char
, the size of wchar_t
may be greater than 1. Consequently, fread()
could attempt to form pointers past the end of wbuf
and use them to assign values to nonexistent elements of the array. Such an attempt results in undefined behavior 109. A likely consequence of this undefined behavior is a buffer overflow. For a discussion of this programming error in the Common Weakness Enumeration database, see CWE-121, "Access of memory location after end of buffer," and CWE-805, "Buffer access with incorrect length value."
#include <stddef.h> #include <stdio.h> void f(FILE *file) { enum { BUFFER_SIZE = 1024 }; wchar_t wbuf[BUFFER_SIZE]; const size_t size = sizeof(*wbuf); const size_t nitems = sizeof(wbuf); size_t nread; nread = fread(wbuf, size, nitems, file); }
Compliant Solution (One Pointer + Two Integers)
This compliant solution correctly computes the maximum number of items for fread()
to read from the file:
#include <stddef.h> #include <stdio.h> void f(FILE *file) { enum { BUFFER_SIZE = 1024 }; wchar_t wbuf[BUFFER_SIZE]; const size_t size = sizeof(*wbuf); const size_t nitems = sizeof(wbuf) / size; size_t nread; nread = fread(wbuf, size, nitems, file); }
Risk Assessment
Depending on the library function called, an attacker may be able to use a heap or stack overflow vulnerability to run arbitrary code.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ARR38-C | High | Likely | Medium | P18 | L1 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
|
| |
Coverity | 6.5 | BUFFER_SIZE | Fully Implemented |
5.0 |
| Can detect violations of this rule with CERT C Rule Pack | |
2024.1 | ABR |
| |
PRQA QA-C | Unable to render {include} The included page could not be found. | 2931 | Fully implemented |
3.1.1 |
|
|
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
CERT vulnerability 720951 describes a vulnerability in OpenSSL versions 1.0.1 through 1.0.1f, popularly known as "Heartbleed". This vulnerability allows a malicious packet fed to a server using OpenSSL to trick that server into returning up to 64 kilobytes of its internal memory. This memory can contain sensitive information, including cryptographic keys, usernames and passwords.
The vulnerable code appears below:
int dtls1_process_heartbeat(SSL *s) { unsigned char *p = &s->s3->rrec.data[0], *pl; unsigned short hbtype; unsigned int payload; unsigned int padding = 16; /* Use minimum padding */ /* Read type and payload length first */ hbtype = *p++; n2s(p, payload); pl = p; // ... if (hbtype == TLS1_HB_REQUEST) { unsigned char *buffer, *bp; int r; /* Allocate memory for the response, size is 1 byte * message type, plus 2 bytes payload length, plus * payload, plus padding */ buffer = OPENSSL_malloc(1 + 2 + payload + padding); bp = buffer; /* Enter response type, length and copy payload */ *bp++ = TLS1_HB_RESPONSE; s2n(payload, bp); memcpy(bp, pl, payload);
This code processes a 'heartbeat' packet from a client. The p
pointer, along with payload
and p1
contain data from this packet. The code allocates a buffer
sufficient to contain payload
bytes, with some overhead, and copies payload
bytes starting at p1
into this buffer, and sends it to the client. Notably absent are any checks that payload
actually indicates the correct size of the memory. Because an attacker can specify an arbitrary value for payload
, she can cause this routine to read and return memory beyond the block allocated to p
.
Related Guidelines
C Secure Coding Standard | API00-C. Functions should validate their parameters ARR01-C. Do not apply the sizeof operator to a pointer when taking the size of an array INT30-C. Ensure that unsigned integer operations do not wrap |
ISO/IEC TS 17961 | Forming invalid pointers by library functions [libptr] |
ISO/IEC TR 24772:2013 | Buffer Boundary Violation (Buffer Overflow) [HCB] |
MITRE CWE
| CWE-119, Failure to constrain operations within the bounds of an allocated memory buffer |
Bibliography
[ISO/IEC TS 17961] | Programming Languages, Their Environments and System Software Interfaces |
Existential Type Crisis : Diagnosis of the OpenSSL Heartbleed Bug |