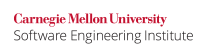
The variable parameters of a variadic function, that is, those that correspond with the position of the ellipsis, are interpreted by the va_arg()
macro. The va_arg()
macro is used to extract the next argument from an initialized argument list within the body of a variadic function implementation. The size of each parameter is determined by the specified type. If the type is inconsistent with the corresponding argument, the behavior is undefined and may result in misinterpreted data or an alignment error (see EXP36-C. Do not convert pointers into more strictly aligned pointer types).
The variable arguments to a variadic function are not checked for type by the compiler. So, the programmer is responsible for ensuring that they are compatible with the corresponding parameter after the default argument promotions:
- Integer arguments of types ranked lower than
int
are promoted toint
, ifint
can hold all the values of that type; otherwise, they are promoted tounsigned int
(the "integer promotions"). - Arguments of type
float
are promoted todouble
.
Noncompliant Code Example (Type Interpretation Error)
The C99 printf()
function is implemented as a variadic function. This noncompliant code example swaps its null-terminated byte string and integer parameters with respect to how they were specified in the format string. Consequently, the integer is interpreted as a pointer to a null-terminated byte string and dereferenced. This will likely cause the program to abnormally terminate. Note that the error_message
pointer is likewise interpreted as an integer.
const char *error_msg = "Error occurred"; /* ... */ printf("%s:%d", 15, error_msg);
Compliant Solution (Type Interpretation Error)
This compliant solution is formatted so that the specifiers are consistent with their parameters.
const char *error_msg = "Error occurred"; /* ... */ printf("%d:%s", 15, error_msg);
As shown, care must be taken to ensure that the arguments passed to a format string function match up with the supplied format string.
Noncompliant Code Example (Type Alignment Error)
In this noncompliant code example, a type long long
integer is incorrectly parsed by the printf()
function with a %d
specifier. This code may result in data truncation or misrepresentation when the value is extracted from the argument list.
long long a = 1; const char msg[] = "Default message"; /* ... */ printf("%d %s", a, msg);
Because a long long
was not interpreted, if the long long
uses more bytes for storage, the subsequent format specifier %s
is unexpectedly offset, causing unknown data to be used instead of the pointer to the message.
Compliant Solution (Type Alignment Error)
This compliant solution adds the length modifier ll
to the %d
format specifier so that the variadic function parser for printf()
extracts the correct number of bytes from the variable argument list for the long long
argument.
long long a = 1; const char msg[] = "Default message"; /* ... */ printf("%lld %s", a, msg);
Risk Assessment
Inconsistent typing in variadic functions can result in abnormal program termination or unintended information disclosure.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL11-C |
high |
probable |
high |
P6 |
L2 |
Automated Detection
GCC Compiler Version 3.4.4 warns about inconsistently typed arguments to formatted output functions when the -Wall
is used.
Compass/ROSE does not currently detect violations of this rule. While the rule in general cannot be automated, due to the difficulty in enforcing contracts between a variadic function and its invokers, it would be fairly easy to enforce type correctness on arguments to the printf()
family of functions.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as DCL11-CPP. Ensure type consistency when using variadic functions.
References
[[ISO/IEC 9899:1999]] Section 6.5.2.2, "Function calls," and Section 7.15, "Variable arguments"
[[ISO/IEC PDTR 24772]] "IHN Type system" and "OTR Subprogram Signature Mismatch"
[[MISRA 04]] Rule 16.1
02. Declarations and Initialization (DCL)