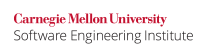
Background
While it used to be common practice to use integers and pointers interchangeably in C (although it was not considered good style), the C99 standard has mandated that pointer to integer and integer to pointer conversions are implementation defined. This means that they are not necessarily portable from one system to the next, and additionally multiple conversions may or may not give the desired behavior.
This means that pointer to integer conversions should generally be avoided, and special care should be taken with NULL and 0 pointers. This recommendation details the proper code for both conversions and null/integer behavior.
Part 1: NULL and 0
If the compiler can successfully identify a '0' in code as being assigned to pointer, then it will convert it to a NULL pointer. This applies to most pointer operations, but it does not apply to particular function calls that do not explicitly specify they will take a pointer. This may lead to a pointer to integer cast, which, as described above, is a bad idea in general, and in this case not even intended.
Non Compliant Code:
execl("/bin/sh", "sh", "-c", "ls", 0);
Code example from the old comp.lang.faq on null pointers (linked below).
From the execl man page for Fedora Linux:
"int execl(const char *path, const char *arg, ...);
...The const char *arg and subsequent ellipses in the execl, execlp, and
execle functions can be thought of as arg0, arg1, ..., argn. Together
they describe a list of one or more pointers to null-terminated
strings that represent the argument list available to the executed
program. The first argument, by convention, should point to the file
name associated with the file being executed. The list of arguments
must be terminated by a NULL pointer."
Therefore, because the type of the '0' argument is not explicitly a pointer, it will be cast to an integer. It is thusly necessary to force 0 to be convert to a pointer by casting.
Compliant Code
execl("/bin/sh", "sh", "-c", "ls", (char *)0);
Because of the cast, this will pass a null pointer. This represents the end of the list of arguments.
Note: Using NULL in place of the 0 will not change the need to cast. The preprocessor will convert the NULLS to 0's and use the same rules for pointer casting as described above.
Part 2: Universal Integer/Pointer Storage
It is recommended to use a union if you need to memory to be accessible as both a pointer and an integer rather than make the cast. Since a union is the size of the largest element and will faithfully represent both as the implementation defines, it will ensure the proper behavior and keep data from being lost.
Non-Compliant Code
unsigned int * chick = 0xcafebabe; ... unsigned int hotchick = chick+1; unsigned int * blinddate = chick;
Compliant Code
union intpoint { unsigned int * pointer; unsigned int number; } intpoint; ... intpoint mydata = 0xcafebabe; ... unsigned int hotchick = mydata.number+1; unsigned int * blinddate = mydata.pointer;
The non-compliant code leads to many possible conversion errors and additionally overflow. All of these are avoided by using a union.
Part 3: Accessing Memory-Mapped Addresses
Note: This is a bad idea. The following is a description of how to properly execute a bad idea.
Often times in low level, kernel, and graphics code you will need to access memory at a specific locations. This means you are going to have to make a literal integer to pointer to conversion! This will make your code non-portable!
The trick here is to convince the compiler you are going to do something bad instead of just assigning a pointer to an integer.
Non-Compliant Code
unsigned int * happyplace = 0xcafebabe;
Because integers are no longer pointers this could have drastic consequences.
Compliant Code
unsigned int * happyplace = (unsigned int *) 0xcafebabe;
The trick here is you are telling the compiler you really do want to do this bad thing. If on your system pointers are typecast to something other than an unsigned int, then you want to use the type they are defined to be instead of unsigned int.
Credits:
- A lot of my ideas and information on NULL and 0 came from the old comp.lang.c FAQ. Read it here
- I used this little tutorial to help me figure out the particulars of unions, as they are not often used. Read it here
- Even more of my ideas came from the more recent comp.lang.c FAQ. Read it here