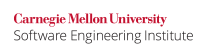
Mutexes are used to protect shared data structures being accessed concurrently. The process that locks the mutex owns it, and the owning process should be the only process to unlock or destroy the mutex. If the mutex is destroyed or unlocked while still in use then there is no more protection of critical sections and shared data.
Non-Compliant Code Example
In this example, a race condition exists between a cleanup and worker process. The cleanup process destroys the lock which it believes is no longer in use. If there is a heavily load on the system, the worker process that held the lock could take longer than expected. If the lock is destroyed before the worker process is done modifying the shared data, the program may exhibit unexpected behavior.
pthread_mutex_t theLock; int data; int cleanupAndFinish() { pthread_mutex_destroy(&theLock); data++; return data; } void worker(int value) { pthread_mutex_lock(&theLock); data += value; pthread_mutex_unlock(&theLock); }
Compliant Solution
This solution requires the cleanup function to acquire the lock before destroying it. Doing it this way ensures that the mutex is only be destroyed by the process that owns it. As always, it is important to check for error conditions, to ensure that the mutex is not already destroyed or otherwise inaccessible before we try to lock it.
Remember that pthread_mutex_destroy()
will acquire all necessary locks.
It shall be safe to destroy an initialized mutex that is unlocked. Attempting to destroy a locked mutex results in undefined behavior.
mutex_t theLock; int data; int cleanupAndFinish() { if(!pthread_mutex_destroy(&theLock)) {/* Handle error */} data++; return data; } void worker(int value) { if(!pthread_mutex_lock(&theLock)) {/* Mutex has already been destroyed */} data += value; if(!pthread_mutex_unlock(&theLock){ {/* Handle unlikely error */} }
Risk Assessment
The risks of ignoring mutex ownership are similar to the risk of not using mutexes at all--a violation of data integrity.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
POS31-C |
2 (medium) |
2 (probable) |
1 (high) |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Linux Programmers Manual entry on mutexes "man mutex"
50. POSIX (POS) POS32-C. Include a mutex when using bit-fields in a multi-threaded environment