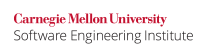
Do not use the same variable name in two scopes where one scope is contained in another. For example,
- No other variable should share the name of a global variable if the other variable is in a subscope of the global variable.
- A block should not declare a variable with the same name as a variable declared in any block that contains it.
Reusing variable names leads to programmer confusion about which variable is being modified. Additionally, if variable names are reused, generally one or both of the variable names are too generic.
Noncompliant Code Example
This noncompliant code example declares the msg
identifier at file scope and reuses the same identifier to declare a character array local to the report_error()
function. The programmer may unintentionally copy the function argument to the locally declared msg
array within the report_error()
function. Depending on the programmer's intention, it either fails to initialize the global variable msg
or allows the local msg
buffer to overflow by using the global value msgsize
as a bounds for the local buffer.
#include <stdio.h> static char msg[100]; static const size_t msgsize = sizeof( msg); void report_error(const char *str) { char msg[80]; snprintf(msg, msgsize, "Error: %s\n", str); /* ... */ } int main(void) { /* ... */ report_error("some error"); return 0; }
Compliant Solution
This compliant solution uses different, more descriptive variable names:
#include <stdio.h> static char message[100]; static const size_t message_size = sizeof( message); void report_error(const char *str) { char msg[80]; snprintf(msg, sizeof( msg), "Error: %s\n", str); /* ... */ } int main(void) { /* ... */ report_error("some error"); return 0; }
When the block is small, the danger of reusing variable names is mitigated by the visibility of the immediate declaration. Even in this case, however, variable name reuse is not desirable. In general, the larger the declarative region of an identifier, the more descriptive and verbose should be the name of the identifier.
By using different variable names globally and locally, the compiler forces the developer to be more precise and descriptive with variable names.
Exceptions
DCL01-EX1: A function argument in a function declaration may clash with a variable in a containing scope provided that when the function is defined, the argument has a name that clashes with no variables in any containing scopes.
extern int name; void f(char *name); /* Declaration: no problem here */ /* ... */ void f(char *arg) { /* Definition: no problem; arg doesn't hide name */ /* Use arg */ }
Risk Assessment
Reusing a variable name in a subscope can lead to unintentionally referencing an incorrect variable.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DCL01-C | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
|
| |
1.2 | CC2.DCL01 | Fully implemented | |
2024.1 | IF_MULTI_DECL |
| |
9.7.1 | 131 S | Fully implemented | |
PRQA QA-C | Unable to render {include} The included page could not be found. | 2547 | Fully implemented |
3.1.1 |
|
|
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
CERT C++ Secure Coding Standard | DCL01-CPP. Do not reuse variable names in subscopes |
MISRA C:2012 | Rule 5.3 (required) |