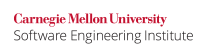
C library functions that make changes to arrays or objects usually take at least two arguments: a pointer to the array or object and an integer indicating the number of elements or bytes to be manipulated. If the arguments are supplied improperly during such a function call, the function may cause the pointer to not point to the object at all or to point past the end of the object, leading to undefined behavior.
Definitions
According to N1579 [1]:
Given an integer expression E, the derived type T of E is determined as follows:
- if E is a
sizeof
expression, then T is the type of the operand of the expression;
- otherwise, if E is an integer identifier, then T is the derived type of the expression last used to store a value in E;
- otherwise, if the derived type of each of E's subexpressions is the same, then T is that type;
- otherwise, the derived type is an unspecified character type compatible with any of char, signed char, and unsigned char.
Consider the following code:
int val; int arr[ARR_SIZE]; size_t c1 = sizeof (val); size_t c2 = sizeof (arr) / sizeof (val); size_t c3 = sizeof (arr) / sizeof (*arr);
The derived type for c1
and c2
is int
, because both subexpressions have the same type. The derived type for c3
is an unspecified character type compatible with any of char
, signed char
, and unsigned char
.
The effective size of a pointer is the size of the object to which it points.
In the following code:
int arr[5]; int *p = arr;
the effective size of the pointer p
is sizeof(arr)
, that is, 5*sizeof(int)
.
The effective type of an object is defined as either its declared type or (if its type isn't declared) the effective type of the value assigned to it.
Consider the following code:
char *p; void *q; q = obj;
In this example, the effective type of p
is char
. The type of q
's type is not declared, but it is later assigned obj
. The effective type of q
is therefore equal to the effective type of obj
.
Standard Library Functions
Here is an incomplete list of C library functions that this rule applies to:
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
*Both functions take more than one size_t
argument. In such cases, the compliant code must be changed according to the purpose of these arguments. For example, in the case of fread()
:
size_t fread(void *ptr, size_t size, size_t count, FILE *stream)
the programmer should make sure the memory block to which ptr
points is of at least (size*count
) bytes.
Description
To guarentee that a library function does not construct an out-of-bounds pointer, programmers must heed the following rules when using functions that operate on pointed-to regions. These rules assume that func
is a function, p
and q
are pointers, and n
is an integer.
- For calls of the form
func(p,n)
, the value ofn
should not be greater than the effective size of the pointer. In situations wheren
is an expression, the effective type of the pointer should be compatible with either the derived type ofn
or unsigned char. - For calls of the form
func(p,q,n)
, the value ofn
should not be greater than the effective size of any of the two pointers (p
andq
). The effective type ofp
should be compatible with the derived type ofn
orunsigned char
whenn
is an expression. Similarly, the effective type ofp
should be compatible with the effective type ofq
orunsigned char
. - For any expression E of the form
T* p = func(n)
, the value ofn
should not be less thansizeof(T)
. Also, the effective type ofT
should be compatible with either the derived type ofn
orunsigned char
.
Noncompliant Code Example
This noncompliant code example assigns a value greater than the size of available memory to n
, which is then passed to memset()
.
void f1(size_t nchars) { char *p = (char *)malloc(nchars); const size_t n = nchars + 1; memset(p, 0, n); /* ... */ }
Compliant Solution
This compliant solution ensures that the value of n
is not greater than the size of the dynamic memory pointed to by the pointer p
:
void f1(size_t nchars, size_t val) { char *p = (char *)malloc(nchars); const size_t n = val; if (nchars < n) { /* Handle Error */ } else { memset(p, 0, n); } /* ... */ }
Noncompliant Code Example
In this noncompliant code example, the effective type of *p
is float
, and the derived type of the expression n
is int
. This is calculated using the first rule from N1579's [1] definition of derived types (see Definitions section). Because n
contains the result of a sizeof
expression, its derived type is equal to the type of the operand, which is int
.
void f2() { const size_t ARR_SIZE = 4; float a[ARR_SIZE]; const size_t n = sizeof(int) * ARR_SIZE; void *p = a; memset(p, 0, n); /* ... */ }
Note: While still noncompliant, this code will have no ill effects on architectures where sizeof(int)
is equal to sizeof(float)
.
Compliant Solution
In this compliant solution, the derived type of n
is also float
.
void f2() { const size_t ARR_SIZE = 4; float a[ARR_SIZE]; const size_t n = sizeof(float) * ARR_SIZE; void *p = a; memset(p, 0, n); /* ... */ }
Noncompliant Code Example
In this noncompliant code example, the size of n
could be greater than the size of *p
. Also, the effective type of *p
(int
) is different than the effective type of *q
(float
).
void f3(int *a) { float b = 3.14; const size_t n = sizeof(*b); void *p = a; void *q = &b; memcpy(p, q, n); /* ... */ }
Note: While still noncompliant, this code will have no ill effects on architectures where sizeof(int)
is equal to sizeof(float)
.
Compliant Solution
This compliant solution ensures that the value of n
is not greater than the minimum of the effective sizes of *p
and *q
and that the effective types of the two pointers are identical (float
).
void f3(float *a, size_t val) { float b = 3.14; const size_t n = val; void *p = a; void *q = &b; if( (n > sizeof(a)) || (n > sizeof(b)) ) { /* Handle error */ } else { memcpy(p, q, n); /* ... */ } }
Noncompliant Code Example
In this noncompliant code example, the value of n
is greater than the size of T
, that is, sizeof(wchar_t)
. But the derived type of expression n
(wchar_t *
) is not the same as the type of T
because its derived type (see Definitions section) will be equal to the type of p
, which is wchar_t*
. The derived type of n
is calculated using the first rule from N1579's [1] definition of derived types (see Definitions section). Because n
here is a sizeof
expression, its derived type is equal to the type of the operand (p
), which is wchar_t *
.
wchar_t *f4() { const wchar_t *p = L"Hello, World!"; const size_t n = sizeof(p) * (wcslen(p) + 1); wchar_t *q = (wchar_t*) malloc(n); return q; }
Compliant Solution
This compliant solution ensures that the derived type of n
(wchar_t
) is the same as the type of T
(wchar_t
) and that the value of n
is not less than the size of T
.
wchar_t *f4() { const wchar_t *p = L"Hello, World!"; const size_t n = sizeof(wchar_t) * (wcslen(p) + 1); wchar_t *q = (wchar_t*) malloc(n); return q; }
Risk Assessment
Depending on the library function called, the attacker may be able to use a heap overflow vulnerability to run arbitrary code. The detection of checks specified in the introduction can be automated, but the remediation has to be manual.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ARR38-C |
high |
likely |
medium |
P18 |
L1 |
Related Guidelines
API00-C. Functions should validate their parameters (https://www.securecoding.cert.org/confluence/display/seccode/API00-C.+Functions+should+validate+their+parameters)
N1579: N1579, Rule 5.34 Forming invalid pointers by library functions
Bibliography
[1] N1579: N1579, Section 4.5