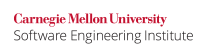
This managed string library was developed in response to the need for a string library that could improve the quality and security of newly developed C language programs while eliminating obstacles to widespread adoption and possible standardization.
The managed string library is based on a dynamic approach in that memory is allocated and reallocated as required. This approach eliminates the possibility of unbounded copies, null-termination errors, and truncation by ensuring there is always adequate space available for the resulting string (including the terminating null character).
A runtime-constraint violation occurs when memory cannot be allocated. In this way, the managed string library accomplishes the goal of succeeding or failing loudly.
The managed string library also provides a mechanism for dealing with data sanitization by (optionally) checking that all characters in a string belong to a predefined set of "safe" characters.
Compliant Solution
This compliant solution illustrates how the managed string library can be used to create a managed string and retrieve a null-terminated byte string from the managed string.
errno_t retValue; char *cstr; /* pointer to null-terminated byte string */ string_m str1 = NULL; if (retValue = strcreate_m(&str1, "hello, world", 0, NULL)) { fprintf(stderr, "Error %d from strcreate_m.\n", retValue); } else { /* retrieve null-terminated byte string and print */ if (retValue = getstr_m(&cstr, str1)) { fprintf(stderr, "error %d from getstr_m.\n", retValue); } printf("(%s)\n", cstr); free(cstr); /* free null-terminated byte string */ }
Note that the calls to fprintf()
and printf()
are C99 standard functions and not managed string functions.
Compliant Solution
This compliant solution illustrates how the SafeStr library can be used to create and manipulate safe strings.
safestr_t string1; safestr_t string2; XXL_TRY_BEGIN { string1 = safestr_create("sample string", 0); string2 = safestr_alloc(14, 0); safestr_copy(&string2, string1); safestr_printf(string2); } XXL_CATCH (SAFESTR_ERROR_OUT_OF_MEMORY) { printf("Insufficient Memory.\n"); } XXL_EXCEPT { printf("Operating failed.\n"); } XXL_TRY_END;
Note that printf()
is a C99 standard function and not a SafeStr string function.
Risk Assessment
String handling functions defined in C99 Section 7.21 and elsewhere are susceptible to common programming errors that can lead to serious, exploitable vulnerabilities. Managed strings, when used properly, can eliminate many of these errors--particularly in new development.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
STR01-A |
3 (high) |
2 (probable) |
1 (high) |
P6 |
L2 |
Examples of vulnerabilities resulting from the violation of this recommendation can be found on the CERT website.
References
[[Burch 06]]
[[CERT 06]]
[[ISO/IEC 9899-1999]] Section 7.21, "String handling <string.h>"
[[Seacord 05a]] Chapter 2, "Strings"