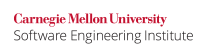
Prevent math errors by carefully bounds-checking before calling functions. In particular, the following domain errors should be prevented by prior bounds-checking:
Function |
Bounds-checking |
---|---|
-1 <= x && x <= 1 |
|
x != 0 || y != 0 |
|
x >= 0 |
|
x != 0 || y > 0 |
|
x >= 0 |
The calling function should take alternative action if these bounds are violated.
acos( x ), asin( x )
Non-Compliant Code Example
This code may produce a domain error if the argument is not in the range [-1, +1].
float x, result; result = acos(x);
Compliant Solution
This code uses bounds checking to ensure there is not a domain error.
float x, result; if ( islessequal(x,-1) || isgreaterequal(x, 1) ){ /* handle domain error */ } result = acos(x);
atan2( y, x )
Non-Compliant Code Example
This code may produce a domain error if both x and y are zero.
float x, y, result; result = atan2(y, x);
Compliant Solution
This code tests the arguments to ensure that there is not a domain error.
float x, y, result; if ( fpclassify(x) == FP_ZERO && fpclassify(y) == FP_ZERO){ /* handle domain error */ } result = atan2(y, x);
log( x ), log10( x )
Non-Compliant Code Example
This code may produce a domain error if x is negative and a range error if x is zero.
float result, x; result = log(x);
Compliant Solution
This code tests the suspect arguments to ensure no domain or range errors are raised.
float result, x; if (islessequal(x, 0)){ /* handle domain and range errors */ } result = log(x);
pow( x, y )
Non-Compliant Code Example
This code may produce a domain error if x is zero and y less than or equal to zero. A range error may also occur if x is zero and y is negative.
float x, y, result; result = pow(x, y);
Compliant Solution
This code tests x and y to ensure that there will be no range or domain errors.
float x, y, result; if (fpclassify(x) == FP_ZERO && islessequal(y, 0)){ /* handle domain error condition */ } result = pow(x, y);
sqrt( x )
Non-Compliant Code Example
This code may produce a domain error if x is negative.
float x, result; result = sqrt(x);
Compliant Solution
This code tests the suspect argument to ensure no domain error is raised.
float x, result; if (isless(x, 0)){ /* handle domain error */ } result = sqrt(x);
Risk Assessment
Failure to properly verify arguments supplied to math functions may result in unexpected results.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FLP32-C |
2 (medium) |
2 (probable) |
2 (medium) |
P8 |
L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 7.12, "Mathematics <math.h>"
[[Plum 91]] Topic 2.10, "conv - conversions and overflow"