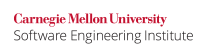
Avoid performing bitwise and arithmetic operations on the same data. In particular, it is frequently the case that bitwise operations are performed on arithmetic values as a form of premature optimization. Bitwise operators include the unary operator ~ and the binary operators <<
, >>
, &
, ^, and |
. Although such operations are valid and will compile, they can reduce code readability. Declaring a variable as containing a numeric value or a bitmap makes the programmer's intentions clearer and the code more maintainable.
Bitmapped types may be defined to further separate bit collections from numeric types. This may make it easier to verify that bitwise operations are only performed on variables that represent bitmaps.
typedef uint32_t bitmap32_t; bitmap32_t bm32 = 0x000007f3; x = (x << 2) | 3; /* shifts in two 1-bits from the right */
The typedef
name uintN_t
designates an unsigned integer type with width N
. Consequently, uint32_t
denotes an unsigned integer type with a width of exactly 32 bits. Bitmaps are normally declared as unsigned.
Non-Compliant Code Example (Left Shift)
In this non-compliant code example, both bit manipulation and arithmetic manipulation are performed on the integer type x
. The result is a (prematurely) optimized statement that assigns 5x + 1
to x
for implementations where integers are represented as two's complement values.
int x = 50; x += (x << 2) + 1;
Although this is a valid manipulation, the result of the shift depends on the underlying representation of the integer type and is consequently implementation-defined. Additionally, the readability of the code is reduced.
Compliant Solution (Left Shift)
In this compliant solution, the assignment statement is modified to reflect the arithmetic nature of x
, resulting in a clearer indication of the programmer's intentions.
int x = 50; x = 5 * x + 1;
A reviewer may now recognize that the operation should be checked for integer overflow. This might not have been apparent in the original, non-compliant code example.
Non-Compliant Code Example (Right Shift)
In this non-compliant code example, the programmer prematurely optimizes code by replacing a division with a right shift.
int x = -50; x >>= 2;
Although this code is likely to perform the division correctly, it is not guaranteed to. If x
has a signed type and a negative value, the operation is implementation-defined and could be implemented as either an arithmetic shift or a logical shift. In the event of a logical shift, if the integer is represented in either one's complement or two's complement form, the most significant bit (which controls the sign in a different way for both representations) will be set to zero. This will cause a once negative number to become a possibly very large positive number.
For example, if the internal representation of x
is 0xFFFF FFCE
(two's complement), an arithmetic shift results in 0xFFFF FFF3
(-13 in two's complement), while a logical shift results in 0x3FFF FFF3
(1 073 741 811 in two's complement).
Compliant Solution (Right Shift)
In this compliant solution, the right shift is replaced by division.
int x = -50; x /= 4;
The resulting value is now more likely to be consistent with the programmer's expectations.
Risk Assessment
Performing bit manipulation and arithmetic operations on the same variable obscures the programmer's intentions and reduces readability. This in turn makes it more difficult for a security auditor or maintainer to determine which checks must be performed to eliminate security flaws and ensure data integrity.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT14-A |
2 (medium) |
1 (unlikely) |
2 (medium) |
P4 |
L3 |
Automated Detection
The LDRA tool suite V 7.6.0 is able to detect violations of this recommendation.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 6.2.6.2, "Integer types"
[[MISRA 04]] Rules 6.4 and 6.5
[[Steele 77]]
INT13-A. Do not assume that a right shift operation is implemented as a logical or an arithmetic shift 04. Integers (INT) INT15-A. Take care when converting from pointer to integer or integer to pointer