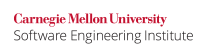
Bitwise shifts include left-shift operations of the form shift-expression <<
additive-expression and right-shift operations of the form shift-expression >>
additive-expression. The integer promotions are performed on the operands, each of which has an integer type. The type of the result is that of the promoted left operand. If the value of the right operand is negative or is greater than or equal to the width of the promoted left operand, the behavior is undefined.
In almost every case, an attempt to shift by a negative number of bits or by more bits than exist in the operand indicates a bug (logic error). This is different than overflow, where there is simply a representational deficiency (see INT32-C. Ensure that operations on signed integers do not result in overflow).
Noncompliant Code Example (Left Shift, Signed Type)
The result of E1 << E2
is E1
left-shifted E2
bit positions; vacated bits are filled with zeros. If E1
has a signed type and nonnegative value and E1 * 2 E2
is representable in the result type, then that is the resulting value; otherwise, the behavior is undefined.
The following code can result in undefined behavior because there is no check to ensure that left and right operands have non-negative values and that the right operand is less than or equal to the width of the promoted left operand.
int si1; int si2; int sresult; sresult = si1 << si2;
Shift operators, and other bitwise operators, should only be used with unsigned integer operands, in accordance with INT13-C. Use bitwise operators only on unsigned operands.
Noncompliant Code Example (Left Shift, Unsigned Type)
The result of E1 << E2
is E1
left-shifted E2
bit positions; vacated bits are filled with zeros. According to C99, if E1
has an unsigned type, the value of the result is E1 * 2 E2
, reduced modulo one more than the maximum value representable in the result type. Although C99 specifies modulo behavior for unsigned integers, unsigned integer overflow frequently results in unexpected values and resultant security vulnerabilities (see INT32-C. Ensure that operations on signed integers do not result in overflow). Consequently, unsigned overflow is generally noncompliant and E1 * 2 E2
must be representable in the result type. Modulo behavior is allowed if the conditions in the exception section are met.
The following code can result in undefined behavior because there is no check to ensure that the right operand is less than or equal to the width of the promoted left operand.
unsigned int ui1; unsigned int ui2; unsigned int uresult; uresult = ui1 << ui2;
Compliant Solution (Left Shift, Unsigned Type)
This compliant solution eliminates the possibility of undefined behavior resulting from a left-shift operation on unsigned integers. Example solutions are provided for the fully compliant case (unsigned overflow is prohibited) and the exceptional case (modulo behavior is allowed).
unsigned int ui1 unsigned int ui2 unsigned int uresult; unsigned int mod1; /* modulo behavior is allowed by exception */ unsigned int mod2; /* modulo behavior is allowed by exception */ /* ... */ if ( (ui2 >= sizeof(unsigned int)*CHAR_BIT) || (ui1 > (UINT_MAX >> ui2))) ) { /* handle error condition */ } else { uresult = ui1 << ui2; } if (mod2 >= sizeof(unsigned int)*CHAR_BIT) { /* handle error condition */ } else { /* modulo behavior is allowed by exception */ uresult = mod1 << mod2; }
Noncompliant Code Example (Right Shift)
The result of E1 >> E2
is E1
right-shifted E2
bit positions. If E1
has an unsigned type or if E1
has a signed type and a nonnegative value, the value of the result is the integral part of the quotient of E1 / 2 E2
. If E1
has a signed type and a negative value, the resulting value is implementation-defined and may be either an arithmetic (signed) shift, as depicted here,
or a logical (unsigned) shift.
This noncompliant code example fails to test whether the right operand is greater than or equal to the width of the promoted left operand, allowing undefined behavior.
unsigned int ui1 = /* initialized using untrusted data */; unsigned int ui2 = /* initialized using untrusted data */; unsigned int uresult; uresult = ui1 >> ui2;
Compliant Solution (Right Shift)
This compliant solution tests the suspect shift operations to guarantee there is no possibility of undefined behavior.
unsigned int ui1 = /* initialized using untrusted data */; unsigned int ui2 = /* initialized using untrusted data */; unsigned int uresult; if (ui2 >= sizeof(unsigned int) * CHAR_BIT) { /* handle error condition */ } else { uresult = ui1 >> ui2; }
Exceptions
INT36-EX1: Unsigned integers can be allowed to exhibit modulo behavior if and only if
- the variable declaration is clearly commented as supporting modulo behavior
- each operation on that integer is also clearly commented as supporting modulo behavior
If the integer exhibiting modulo behavior contributes to the value of an integer not marked as exhibiting modulo behavior, the resulting integer must obey this rule.
Risk Assessment
Improper range checking can lead to buffer overflows and the execution of arbitrary code by an attacker.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT34-C |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
Fortify SCA Version 5.0 with CERT C Rule Pack can detect violations of this rule.
Compass/ROSE can detect violations of this rule. Unsigned operands are detected when checking for INT13-C. Use bitwise operators only on unsigned operands.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
A test program for this rule is available.
[[Dowd 06]] Chapter 6, "C Language Issues"
[[ISO/IEC 9899:1999]] Section 6.5.7, "Bitwise shift operators"
[[ISO/IEC PDTR 24772]] "XYY Wrap-around Error"
[[Seacord 05a]] Chapter 5, "Integers"
[[Viega 05]] Section 5.2.7, "Integer overflow"
[[ISO/IEC 03]] Section 6.5.7, "Bitwise shift operators"
INT33-C. Ensure that division and modulo operations do not result in divide-by-zero errors 04. Integers (INT) INT35-C. Evaluate integer expressions in a larger size before comparing or assigning to that size