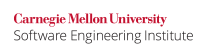
C99 Section 7.12.1 defines two types of errors that relate specifically to math functions in math.h
[[ISO/IEC 9899:1999]]:
a domain error occurs if an input argument is outside the domain over which the mathematical function is defined.
a range error occurs if the mathematical result of the function cannot be represented in an object of the specified type, due to extreme magnitude.
An example of a domain error is the square root of a negative number, such as sqrt(-1.0)
, which has no meaning in real arithmetic. Similarly, ten raised to the one-millionth power, pow(10., 1e6)
, likely cannot be represented in an implementation's floating point representation and consequently constitutes a range error.
In both cases, the function will return some value, but the value returned is not the correct result of the computation.
Domain errors can be prevented by carefully bounds checking the arguments before calling functions, and taking alternative action if the bounds are violated.
Range errors usually can not be prevented, as they are dependent on the implementation of floating-point numbers, as well as the function being applied. Instead of preventing range errors, one should attempt to detect them and take alternative action if a range error occurs.
The following table lists standard mathematical functions, along with any checks that should be performed on their domain, and indicates if they also throw range errors, as reported by [ISO/IEC 9899:1999]. If a function has domain checks, one should check its input values, and if a function throws range errors, one should detect if a range error occurs. The standard math functions not on this table, such as atan()
have no domain restrictions and do not throw range errors.
Function |
Domain |
Range |
---|---|---|
|
-1 <= x && x <= 1 |
no |
|
x != 0 || y != 0 |
no |
|
x >= 1 |
no |
|
-1 < x && x < 1 |
no |
none |
yes |
|
|
none |
yes |
|
none |
yes |
|
x > 0 |
no |
|
x > -1 |
no |
|
x != 0 |
yes |
|
none |
yes |
|
none |
yes |
x > 0 || (x == 0 && y > 0) || |
yes |
|
x >= 0 |
no |
|
|
none |
yes |
|
(x == 0) || |
yes |
|
no |
yes |
|
y != 0 |
no |
|
none |
yes |
|
none |
yes |
|
none |
yes |
Domain Checking
The most reliable way to handle domain errors to prevent them by checking arguments beforehand, as in the following template:
if (/* arguments will cause a domain error */) { /* handle domain error */ } else { /* perform computation */ }
Range Checking
Range errors can usually not be prevented, so the most reliable way to handle range errors is to detect when they have occurred and act accordingly. The following approach uses C99 standard functions for floating point errors.
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; /* call the function */ #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* handle range error */ } #endif
See FLP03-C. Detect and handle floating point errors for more details on how to detect floating point errors.
Error Checking and Detection
The exact treatment of error conditions from math functions is quite complicated. C99 Section 7.12.1 defines the following behavior for floating point overflow [[ISO/IEC 9899:1999]]
A floating result overflows if the magnitude of the mathematical result is finite but so large that the mathematical result cannot be represented without extraordinary roundoff error in an object of the specified type. If a floating result overflows and default rounding is in effect, or if the mathematical result is an exact infinity from finite arguments (for example
log(0.0)
), then the function returns the value of the macroHUGE_VAL
,HUGE_VALF
, orHUGE_VALL
according to the return type, with the same sign as the correct value of the function; if the integer expressionmath_errhandling & MATH_ERRNO
is nonzero, the integer expressionerrno
acquires the valueERANGE
; if the integer expressionmath_errhandling & MATH_ERREXCEPT
is nonzero, the ''divide-by-zero'' floating-point exception is raised if the mathematical result is an exact infinity and the ''overflow'' floating-point exception is raised otherwise.
It is best not to check for errors by comparing the returned value against HUGE_VAL
or 0
for several reasons:
- These are in general valid (albeit unlikely) data values.
- Making such tests requires detailed knowledge of the various error returns for each math function.
- There are three different possibilities,
-HUGE_VAL
,0
, andHUGE_VAL
, and you must know which are possible in each case. - Different versions of the library have differed in their error-return behavior.
It is also difficult to check for math errors using errno
because an implementation might not set it. For real functions, the programmer can tell whether the implementation sets errno
by checking whether math_errhandling & MATH_ERRNO
is nonzero. For complex functions, the C99 Section 7.3.2 simply states "an implementation may set errno
but is not required to" [[ISO/IEC 9899:1999]].
Implementation Details
System V Interface Definition, Third Edition
The System V Interface Definition, Third Edition (SVID3) provides more control over the treatment of errors in the math library. The user can provide a function named matherr
that is invoked if errors occur in a math function. This function can print diagnostics, terminate the execution, or specify the desired return-value. The matherr()
function has not been adopted by C99, so its use is not generally portable.
sqrt(x)
Noncompliant Code Example
The following noncompliant code determines the square root of x
double x; double result; result = sqrt(x);
However, this code may produce a domain error if x
is negative.
Compliant Solution
Since this function has domain errors but no range errors, one can use bounds checking to prevent domain errors.
double x; double result; if (isless(x, 0)) { /* handle domain error */ } result = sqrt(x);
cosh(x), sinh(x)
Noncompliant Code Example
The following noncompliant code determines the hyperbolic cosine of x
.
double x; double result; result = cosh(x);
However, this code may produce a range error if x
has a very large magnitude.
Compliant Solution
Since this function has no domain errors but may have range errors, one must detect a range error and act accordingly.
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; double x; double result; result = sinh(x); #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* handle range error */ } #endif
pow(x, y)
Noncompliant Code Example
The following noncompliant code raises x
to the power of y
.
double x; double y; double result; result = pow(x, y);
However, this code may produce a domain error if x
is negative and y
is not an integer, or if x
is zero and y
is zero. A domain error or range error may occur if x
is zero and y
is negative, and a range error may occur if the result cannot be represented as a double
.
Noncompliant Code Example
Since the pow()
function can produce both domain errors and range errors, we must first check that x
and y
lie within the proper domain. We must also detect if a range error occurs, and act accordingly.
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; /* call the function */ double x; double y; double result; if (((x == 0.f) && islessequal(y, 0)) || (isless(x, 0))) { /* handle domain error */ } result = pow(x, y); #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* handle range error */ } #endif
Risk Assessment
Failure to properly verify arguments supplied to math functions may result in unexpected results.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FLP32-C |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
Fortify SCA Version 5.0 with CERT C Rule Pack can detect violations of this rule.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]] Section 7.3, "Complex arithmetic <complex.h
>", and Section 7.12, "Mathematics <math.h
>"
[[MITRE 07]] CWE ID 682, "Incorrect Calculation"
[[Plum 85]] Rule 2-2
[[Plum 89]] Topic 2.10, "conv - conversions and overflow"
FLP31-C. Do not call functions expecting real values with complex values 05. Floating Point (FLP) FLP33-C. Convert integers to floating point for floating point operations