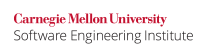
Under Construction
This is new rule and not yet ready for review.
It is only appropriate to add or subtract integers to pointers to an element of an array object.
If the pointer operand points to an element of an array object, and the array is large enough, the result points to an element offset from the original element such that the difference of the subscripts of the resulting and original array elements equals the integer expression.
Non-Compliant Code Example
In this non-compliant code example, the programmer tried to access elements of the structure using pointer arithmetic. This is dangerous because fields in a structure are not guaranteed to be contiguous.
struct numbers { int num1; int num2; /* ... */ int num64; }; int sum_numbers(struct numbers *numb){ int total = 0; int *numb_ptr; for (numb_ptr = &numb->num1; numb_ptr <= &numb->num64; numb_ptr++) { total += *(numb_ptr); } return total; } int main(void) { struct numbers my_numbers = { 1, 2, /* ... */ 64 }; sum_numbers(&my_numbers); return 0; }
Compliant Solution
It is possible to use the ->
operator to deference each element of the structure, for example:
total = numb->num1 + numb->num2 + /* ... */ numb->num64;
However, this is likely to be exactly the sort of painful experience the programmer who wrote the non-compliant coding example was trying to avoid.
A better solution is to use an array, as in this compliant solution:
int sum_numbers(int *numb, size_t dim) { int total = 0; int *numb_ptr; for (numb_ptr = numb; numb_ptr < numb + dim; numb_ptr++) { total += *(numb_ptr); } return total; } int main(void) { int my_numbers[] = { 1, 2, /* ... */ 64 }; sum_numbers(my_numbers, sizeof(my_numbers)/sizeof(my_numbers[0])); return 0; }
Arrays elements are guaranteed to be contiguous in memory, so this solution is completely portable.
Risk Assessment
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ARR37-C |
2 (medium) |
2 (probable) |
1 (high) |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Banahan 03]] Section 5.3, "Pointers," and Section 5.7, "Expressions involving pointers"
[[ISO/IEC 9899-1999]] Section 6.5.6, "Additive operators"
[[VU#162289]]