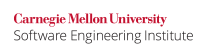
When two pointers are subtracted, both must point to elements of the same array object, or one past the last element of the array object; the result is the difference of the subscripts of the two array elements. This restriction exists because pointer subtraction in C produces the number of objects between the two pointers, not the number of bytes.
Similarly comparing pointers can tell you the relative positions of the pointers in term of each other. Subtracting or comparing pointers that do not refer to the same array can result in erroneous behavior.
It is acceptable to compare two member pointers within a single struct
object, suitably cast, because any object can be treated as an array of unsigned char
.
Non-Compliant Code Example
In this non-compliant code example pointer subtraction is used to determine how many free elements are left in the nums
array.
int nums[SIZE]; char *strings[SIZE]; int *next_num_ptr = nums; int free_bytes; /* increment next_num_ptr as array fills */ free_bytes = strings - (char *)next_num_ptr;
The first incorrect assumption is that nums
and strings arrays are necessarily contingent in memory. The second is that free_bytes
is the number of bytes available. The subtraction will return the number of elements between next_num_ptr
and strings.
Compliant Solution
In this compliant solution, the number of free elements is kept as a counter and adjusted on every array operation. It is also calculated in terms of free elements instead if bytes. This prevents further mathematical errors.
int nums[SIZE]; char *strings[SIZE]; int *next_num_ptr = nums; int free_bytes; /* increment next_num_ptr as array fills */ free_bytes = (next_num_ptr - nums) * sizeof(int);
Risk Assessment
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ARR36-C |
2 (medium) |
2 (probable) |
2 (medium) |
P8 |
L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Banahan 03]] Section 5.3, "Pointers," and Section 5.7, "Expressions involving pointers"
[[ISO/IEC 9899-1999]] Section 6.5.6, "Additive operators"
ARR35-C. Do not allow loops to iterate beyond the end of an array 06. Arrays (ARR)