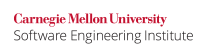
Many functions require the allocation of multiple objects. Failing and returning somewhere in the middle of this function without freeing all of the allocated memory could produce a memory leak. It is a common error to forget to free one (or all) of the objects in this manner, so a goto-chain is the simplest and cleanest way to organize exits when order is preserved.
Noncompliant Code Exampleint do_something(void){
// ... definitions ,,,
obj1 = malloc(...);
if (!obj1)
obj2 = malloc(...);
if (!obj2)
obj3 = malloc(...);
if (!obj3)
// ... more code ...
}
Compliant Solutionint do_something(void){
// ... definitions ,,,
obj1 = malloc(...);
if (!obj1)
obj2 = malloc(...);
if (!obj2)
obj3 = malloc(...);
if (!obj3)
// ... more code ...
FAIL_OBJ3:
free(obj2);
FAIL_OBJ2:
free(obj1);
FAIL_OBJ1:
return -1;
}
This code is guaranteed to clean up properly whenever an allocation fails. It is cleaner and prevents rewriting of similar code upon every function error.