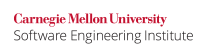
Description
According to C standard - 6.2.5 Types,
C library functions that make changes to arrays or objects usually take at least two arguments: i.) a pointer to the array/object ii.) an integer indicating the number of elements or bytes to be manipulated. If the arguments are supplied improperly during such a function call, the function may cause the pointer to not point to the object at all or point past the end of the object. This would lead to undefined behavior.
To make sure that this does not happen, programmers must keep in mind the following rules when using such functions:
- For func (p,n), where 'p' is the pointer, 'n' is the integer and 'func' is the library function, the value of ânâ should not be greater than the effective size of the pointer. Also, the effective type of the pointer should be compatible with either the derived type of 'n' or unsigned char.
- For func (p,q, n), where 'p' and 'q' are both pointers, 'n' is the integer and 'func' is the library function, the value of ânâ should not be greater than the effective size of any of the two pointers ('p' and 'q'). The effective type of the 'p' should be compatible with the derived type of 'n' or unsigned char. Similarly, the effective type of the 'p' should be compatible with the effective type of 'q' or unsigned char.
- For expression E of the form: T* q = func (n), where 'func' is a memory allocation function, the value of 'n' should not be less than sizeof (T). Also, the effective type of 'T' should be compatible with either the derived type of 'n' or unsigned char.
Noncompliant Code Example
This noncompliant code example assigns a value greater than the size of dynamic memory to 'n' which is then passed to the memset().
void f1 (size_t nchars) { char *p = (char *)malloc(nchars); const size_t n = nchars + 1; memset(p, 0, n); /* More program code */ }
Compliant Solution
This compliant solution makes sure that the value of 'n' is not greater the size of the dynamic memory pointed to by the pointer 'p':
void f1 (size_t nchars, size_t val) { char *p = (char *)malloc(nchars); const size_t n = val; if (nchars < n) { Â Â Â Â /* Handle Error */ } else { memset(p, 0, n); } }
Noncompliant Code Example
In noncompliant code example the effective type of *p is float while the derived type of the expression 'n' is int.
void f2() { const size_t ARR_SIZE = 4; float a[ARR_SIZE]; const size_t n= sizeof(int) * ARR_SIZE; void *p = a; memset(p, 0, n); /* More program code */ }
Note: A possibility of this code being safe would be on architectures where sizeof (int) is equal to sizeof (float).
Compliant Solution
The derived type of 'n' in this solution is also float.
void f2() { const size_t ARR_SIZE = 4; float a[ARR_SIZE]; const size_t n= sizeof(float) * ARR_SIZE; void *p = a; memset(p, 0, n); /* More program code */ }
Noncompliant Code Example
In this noncompliant code example, the size of 'n' could be greater than the size of *p. Also, the effective type of *p (int) is not same as the effective type of *q (float).
void f3(int *a) { float b = 3.14; const size_t n = sizeof(*b); void *p = a; void *q = &b; memcpy(p, q, n); /* More program code */ }
Note: A possibility of this code being safe would be on architectures where sizeof (*int) is equal to sizeof (*float).
Compliant Solution
This compliant solution makes sure that the of 'n' is not greater the the minimum of effective sizes of *p and *q and the effective types of the two pointers is also same (float).
void f3(float *a, size_t val) { float b = 3.14; const size_t n = val; void *p = a; void *q = &b; if( (n > sizeof(a)) || (n > sizeof(b)) ) { /* Handle error */ } else { memcpy(p, q, n); /* More program code */ } }
Noncompliant Code Example
In this noncompliant code example, the value of 'n' is greater than the size of 'T' i.e. sizeof (wchar_t). But, the derived type of expression 'n' (wchar_t *) is not same as the type of 'T' i.e. wchar_t.
wchar_t *f7() { const wchar_t *p = L"Hello, World!"; const size_t n = sizeof(p) * (wcslen(p) + 1); wchar_t *q = (wchar_t *)malloc(n); return q; }
Compliant Solution
This compliant solution makes sure that the derived type of 'n' (wchar_t) is same as the type of 'T' (wchar_t). Also, the value of 'n' is not less than the size of 'T'.
wchar_t *f7() { const wchar_t *p = L"Hello, World!"; const size_t n = sizeof(wchar_t) * (wcslen(p) + 1); wchar_t *q = (wchar_t *)malloc(n); return q; }
Given below is a non-exhaustive list of library functions to which the above rules can apply:
memcpy() |
memmove() |
memset() |
|
wmemcpy() |
wmemmove() |
strftime() |
|
calloc() |
malloc() |
realloc() |
|
strncpy() |
swprintf() |
vswprintf() |
|
wcsncpy() |
strxfrm() |
snprintf() |
|
vsnprintf() |
fwrite() * |
fread() * |
|
* - both the functions take more than one size_t argument. In such cases, the compliant code will have to be changed according to the purpose of these arguments. For example in the case of fread():
size_t fread ( void *ptr, size_t size, size_t count, FILE * stream)
the programmer should make sure that the memory block to which 'ptr' points is of atleast size*count bytes.
Risk Assessment
Depending on the library function called, the attacker may be able to use a heap overflow vulnerability to run arbitrary code. The detection of checks specified in description can be automated but the remediation has to be manual.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ARR38-C |
high |
likely |
medium |
P18 |
L1 |
Related Guidelines
[seccode:API00-C. Functions should validate their parameters]
WG14 Document: N1579 - Rule 5.34 Forming Invalid pointers by library functions.
Bibliography
WG14 Document: N1579 - Rule 5.34 Forming Invalid pointers by library functions.