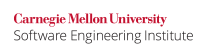
Do not use the same variable name in two scopes where one scope is contained in another. Examples include
- No other variable should share the name of a global variable if the other value is in a subscope of the global variable.
- A block should not declare a variable with the same name as a variable declared in any block that contains it.
Reusing variable names leads to programmer confusion about which variable is being modified. Additionally, if variable names are reused, generally one or both of the variable names are too generic.
Noncompliant Code Example
This noncompliant code example declares the msg
identifier at the start of the compilation unit (with file scope) and reuses the same identifier to declare a character array local to the report_error()
function. Consequently, the programmer unintentionally copies a string to the locally declared msg
array within the report_error()
function, failing to initialize the assign global variable and resulting in a potential buffer overflow.
char msg[100]; void report_error(const char *error_msg) { char msg[80]; /* ... */ strncpy(msg, error_msg, sizeof(msg)); return; } int main(void) { char error_msg[80]; /* ... */ report_error(error_msg); /* ... */ }
Compliant Solution
This compliant solution uses different, more descriptive variable names.
char system_msg[100]; void report_error(const char *error_msg) { char default_msg[80]; /* ... */ if (error_msg) strncpy(system_msg, error_msg, sizeof(system_msg)); else strncpy(system_msg, default_msg, sizeof(system_msg)); return; } int main(void) { char error_msg[80]; /* ... */ report_error(error_msg); /* ... */ }
When the block is small, the danger of reusing variable names is mitigated by the visibility of the immediate declaration. Even in this case, however, variable name reuse is not desirable.
By using different variable names globally and locally, the compiler forces the developer to be more precise and descriptive with variable names.
Risk Assessment
Reusing a variable name in a subscope can lead to unintentionally referencing an incorrect variable.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL01-C |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
The LDRA tool suite Version 7.6.0 can detect violations of this recommendation.
Splint Version 3.1.1 can detect violations of this recommendation.
Compass / ROSE can detect violations of this recommendation.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]] Section 5.2.4.1, "Translation limits"
[[ISO/IEC PDTR 24772]] "BRS Leveraging human experience" and "YOW Identifier name reuse"
[[MISRA 04]] Rule 5.2