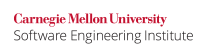
Flexible array members are a special type of array where the last element of a structure with more than one named member has an incomplete array type; that is, the size of the array is not specified explicitly within the structure. A variety of different syntaxes have been used for declaring flexible array members. For C99-compliant implementations, use the syntax guaranteed valid by C99 [[ISO/IEC 9899:1999]].
Non-Compliant Code Example
In this non-compliant code, an array of size 1 is declared, but when the structure itself is instantiated, the size computed for malloc()
is modified to account for the actual size of the dynamic array. This is the syntax used by ISO C89.
struct flexArrayStruct { int num; int data[1]; }; /* ... */ size_t array_size; size_t i; /* initialize array_size */ /* space is allocated for the struct */ struct flexArrayStruct *structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * (array_size - 1)); if (structP == NULL) { /* handle malloc failure */ } structP->num = 0; /* access data[] as if it had been allocated * as data[array_size] */ for (i = 0; i < array_size; i++) { structP->data[i] = 1; }
The problem with this code is that the only member that is guaranteed to be valid, by strict C99 definition, is structP->data[0]
. Consequently, for all i > 0
, the results of the assignment are undefined.
Implementation Details
The non-compliant example may be the only alternative for compilers that do not yet implement the C99 syntax. Microsoft Visual Studio 2005 does not implement the C99 syntax.
Compliant Solution
This compliant solution uses the flexible array member to achieve a dynamically sized structure.
struct flexArrayStruct{ int num; int data[]; }; /* ... */ size_t array_size; size_t i; /* initialize array_size */ /* Space is allocated for the struct */ struct flexArrayStruct *structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (structP == NULL) { /* handle malloc failure */ } structP->num = 0; /* Access data[] as if it had been allocated * as data[array_size] */ for (i = 0; i < array_size; i++) { structP->data[i] = 1; }
This compliant solution allows the structure to be treated as if it had declared the member data[]
to be data[array_size]
in a manner that conforms to the C99 standard.
However, some restrictions apply:
- The incomplete array type must be the last element within the structure.
- There cannot be an array of structures that contain flexible array members.
- Structures that contain a flexible array member cannot be used as a member in the middle of another structure.
- The
sizeof
operator cannot be applied to a flexible array.
Automated Detection
Compass / ROSE detects violations of this rule by searching for structs where the last element is an array, with a small index (0 or 1).
Risk Assessment
Failing to use the correct syntax can result in undefined behavior, although the incorrect syntax will work on most implementations.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MEM33-C |
low |
unlikely |
low |
P3 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]] Section 6.7.2.1, "Structure and union specifiers"
[[McCluskey 01]] ;login:, July 2001, Volume 26, Number 4
MEM32-C. Detect and handle memory allocation errors 08. Memory Management (MEM)